长连接变成短链接,短链接跳转成长连接,起服务在浏览器运营起来
时间: 2023-11-17 13:05:29 浏览: 36
实现长链接变成短链接,短链接跳转成长链接需要使用URL Shortener服务。下面是一个简单的Java代码示例,使用Bitly将长链接转换为短链接:
```java
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import java.net.URLEncoder;
public class URLShortener {
public static void main(String[] args) {
String longUrl = "http://example.com/very-long-url";
String accessToken = "YOUR_ACCESS_TOKEN";
try {
URL url = new URL("https://api-ssl.bitly.com/v4/shorten");
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("POST");
connection.setRequestProperty("Authorization", "Bearer " + accessToken);
connection.setRequestProperty("Content-Type", "application/json");
String postData = "{\"long_url\": \"" + longUrl + "\"}";
connection.setDoOutput(true);
connection.getOutputStream().write(postData.getBytes("UTF-8"));
BufferedReader reader = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String response = reader.readLine();
// Parse the JSON response to get the short URL
String shortUrl = response.substring(response.indexOf("\"link\": \"") + 9, response.indexOf("\","));
System.out.println("Short URL: " + shortUrl);
reader.close();
connection.disconnect();
} catch (Exception e) {
System.err.println("Error: " + e.getMessage());
}
}
}
```
要将短链接跳转到长链接,可以使用Java的HttpURLConnection类。下面是一个简单的示例:
```java
import java.net.HttpURLConnection;
import java.net.URL;
public class URLRedirector {
public static void main(String[] args) {
String shortUrl = "http://bit.ly/abc123";
String accessToken = "YOUR_ACCESS_TOKEN";
try {
URL url = new URL("https://api-ssl.bitly.com/v4/expand?shortUrl=" + URLEncoder.encode(shortUrl, "UTF-8"));
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("GET");
connection.setRequestProperty("Authorization", "Bearer " + accessToken);
BufferedReader reader = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String response = reader.readLine();
// Parse the JSON response to get the long URL
String longUrl = response.substring(response.indexOf("\"long_url\": \"") + 13, response.indexOf("\","));
System.out.println("Long URL: " + longUrl);
reader.close();
connection.disconnect();
} catch (Exception e) {
System.err.println("Error: " + e.getMessage());
}
}
}
```
要在浏览器中运行服务,可以使用Java的HttpServer类。下面是一个简单的示例:
```java
import com.sun.net.httpserver.HttpExchange;
import com.sun.net.httpserver.HttpHandler;
import com.sun.net.httpserver.HttpServer;
import java.io.IOException;
import java.io.OutputStream;
import java.net.InetSocketAddress;
public class SimpleHttpServer {
public static void main(String[] args) throws Exception {
HttpServer server = HttpServer.create(new InetSocketAddress(8000), 0);
server.createContext("/hello", new HelloHandler());
server.setExecutor(null);
server.start();
System.out.println("Server started on port 8000");
}
static class HelloHandler implements HttpHandler {
@Override
public void handle(HttpExchange t) throws IOException {
String response = "Hello, world!";
t.sendResponseHeaders(200, response.length());
OutputStream os = t.getResponseBody();
os.write(response.getBytes());
os.close();
}
}
}
```
在浏览器中访问http://localhost:8000/hello即可看到"Hello, world!"的输出。
相关推荐
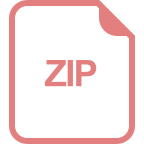





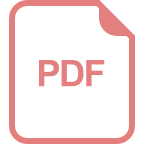
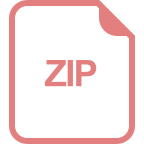
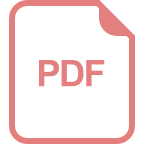
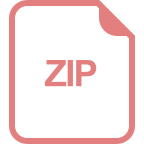
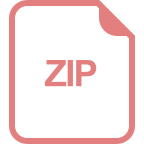
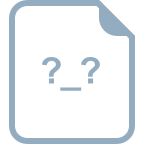
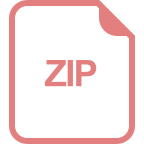
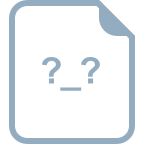