springboot如何调用串口
时间: 2023-10-20 09:07:45 浏览: 137
Spring Boot本身并不直接支持串口通信,需要借助第三方库来实现串口通信。常用的Java串口通信库有rxtx和jssc,这里以jssc为例介绍如何在Spring Boot中调用串口。
1. 添加依赖
在pom.xml文件中添加jssc的依赖:
```xml
<dependency>
<groupId>com.fazecast</groupId>
<artifactId>jSerialComm</artifactId>
<version>2.6.2</version>
</dependency>
```
2. 编写串口通信代码
```java
import jssc.SerialPort;
import jssc.SerialPortException;
import org.springframework.stereotype.Component;
@Component
public class SerialPortService {
private SerialPort serialPort;
public void open(String port, int baudRate) throws SerialPortException {
serialPort = new SerialPort(port);
serialPort.openPort();
serialPort.setParams(baudRate, SerialPort.DATABITS_8, SerialPort.STOPBITS_1, SerialPort.PARITY_NONE);
}
public void close() throws SerialPortException {
serialPort.closePort();
}
public void write(byte[] data) throws SerialPortException {
serialPort.writeBytes(data);
}
public byte[] read() throws SerialPortException {
return serialPort.readBytes();
}
}
```
3. 在Spring Boot中使用串口通信
可以在Controller中注入SerialPortService来使用串口通信功能,例如:
```java
import jssc.SerialPortException;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class SerialPortController {
@Autowired
private SerialPortService serialPortService;
@GetMapping("/serialport")
public String serialPort() throws SerialPortException {
// 打开串口
serialPortService.open("COM1", 9600);
// 发送数据
serialPortService.write("Hello".getBytes());
// 接收数据
byte[] data = serialPortService.read();
// 关闭串口
serialPortService.close();
return new String(data);
}
}
```
在上述代码中,SerialPortController中注入了SerialPortService,通过调用SerialPortService的方法实现串口通信。在实际使用中,需要根据具体的串口设置来配置串口参数,例如串口名称、波特率、数据位、停止位和校验位等。
阅读全文
相关推荐

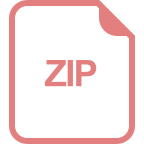


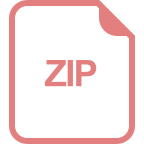
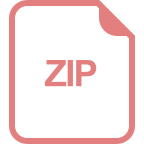
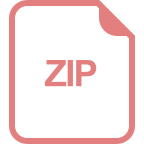
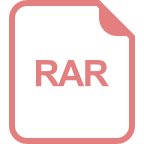
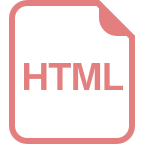
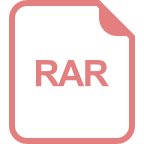
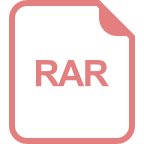
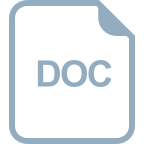
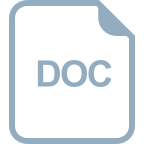
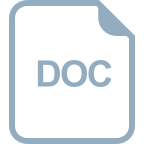
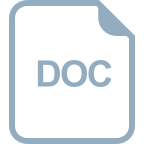
