python入侵外星人200行代码小游戏
时间: 2023-11-03 19:55:18 浏览: 50
以下是一个简单的 Python 小游戏,名为“入侵外星人”。这个游戏的目标是射击下降的外星人并得分。游戏有 3 层外星人,每层外星人的移动速度不同,游戏难度逐渐增加。
```python
import pygame
import sys
from random import randint
pygame.init()
# 游戏设置
width = 800
height = 600
screen = pygame.display.set_mode((width, height))
pygame.display.set_caption("Alien Invasion")
clock = pygame.time.Clock()
# 颜色设置
white = (255, 255, 255)
black = (0, 0, 0)
# 字体设置
font = pygame.font.Font(None, 36)
# 外星人设置
alien_width = 50
alien_height = 50
alien_speed = 5
alien_color = (randint(0, 255), randint(0, 255), randint(0, 255))
# 玩家设置
player_width = 50
player_height = 50
player_speed = 10
player_color = (0, 255, 0)
# 子弹设置
bullet_width = 5
bullet_height = 20
bullet_speed = 10
bullet_color = (255, 0, 0)
# 游戏状态
game_over = False
score = 0
# 外星人生成函数
def generate_aliens(num_rows, num_cols):
aliens = []
for row in range(num_rows):
for col in range(num_cols):
alien = {
"x": col * alien_width,
"y": row * alien_height,
"color": (randint(0, 255), randint(0, 255), randint(0, 255))
}
aliens.append(alien)
return aliens
# 子弹生成函数
def generate_bullet(player_x, player_y):
bullet = {
"x": player_x + player_width / 2 - bullet_width / 2,
"y": player_y - bullet_height,
}
return bullet
# 碰撞检测函数
def collide(rect1, rect2):
if (rect1["x"] < rect2["x"] + rect2["width"] and
rect1["x"] + rect1["width"] > rect2["x"] and
rect1["y"] < rect2["y"] + rect2["height"] and
rect1["y"] + rect1["height"] > rect2["y"]):
return True
return False
# 游戏循环
def game_loop():
global game_over, score
# 外星人生成
aliens = generate_aliens(3, 10)
# 玩家初始化
player_x = width / 2 - player_width / 2
player_y = height - player_height
player_speed_x = 0
# 子弹列表初始化
bullets = []
while not game_over:
# 事件循环
for event in pygame.event.get():
if event.type == pygame.QUIT:
sys.exit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
player_speed_x = -player_speed
elif event.key == pygame.K_RIGHT:
player_speed_x = player_speed
elif event.key == pygame.K_SPACE:
bullet = generate_bullet(player_x, player_y)
bullets.append(bullet)
elif event.type == pygame.KEYUP:
if event.key == pygame.K_LEFT or event.key == pygame.K_RIGHT:
player_speed_x = 0
# 碰撞检测
for bullet in bullets:
for alien in aliens:
if collide(bullet, alien):
bullets.remove(bullet)
aliens.remove(alien)
score += 10
for alien in aliens:
if collide(alien, {"x": player_x, "y": player_y, "width": player_width, "height": player_height}):
game_over = True
# 外星人移动
for alien in aliens:
alien["y"] += alien_speed
if alien["y"] >= height:
game_over = True
# 玩家移动
player_x += player_speed_x
if player_x < 0:
player_x = 0
elif player_x > width - player_width:
player_x = width - player_width
# 子弹移动
for bullet in bullets:
bullet["y"] -= bullet_speed
if bullet["y"] < 0:
bullets.remove(bullet)
# 绘制画面
screen.fill(black)
for alien in aliens:
pygame.draw.rect(screen, alien["color"], [alien["x"], alien["y"], alien_width, alien_height])
pygame.draw.rect(screen, player_color, [player_x, player_y, player_width, player_height])
for bullet in bullets:
pygame.draw.rect(screen, bullet_color, [bullet["x"], bullet["y"], bullet_width, bullet_height])
text = font.render(f"Score: {score}", True, white)
screen.blit(text, (10, 10))
pygame.display.update()
# 控制游戏速度
clock.tick(30)
# 游戏结束
screen.fill(black)
text = font.render("Game Over", True, white)
screen.blit(text, (width / 2 - 50, height / 2 - 18))
text = font.render(f"Score: {score}", True, white)
screen.blit(text, (width / 2 - 50, height / 2 + 18))
pygame.display.update()
pygame.time.delay(2000)
# 启动游戏
game_loop()
```
这个游戏使用了 Pygame 库来实现图形界面和事件响应。游戏界面中有外星人、玩家和子弹三种元素,玩家可以通过键盘控制玩家的移动和子弹的射击,同时需要注意外星人的攻击。游戏难度逐步增加,游戏难度越来越高。
相关推荐
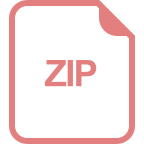
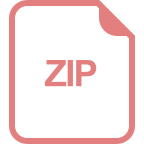












