nestjs typeorm 注册用户 验证登录功能
时间: 2023-07-22 12:23:06 浏览: 204
首先,您需要安装 `@nestjs/typeorm` 和 `bcryptjs` 模块,以便在 NestJS 中使用 TypeORM 和密码哈希。
接下来,您可以创建一个 `User` 实体类,以便在数据库中存储用户信息。例如:
```typescript
import { Entity, Column, PrimaryGeneratedColumn } from 'typeorm';
@Entity()
export class User {
@PrimaryGeneratedColumn()
id: number;
@Column({ unique: true })
username: string;
@Column()
password: string;
}
```
然后,您可以创建一个 `AuthService` 服务,其中包含注册用户和验证登录的方法。例如:
```typescript
import { Injectable } from '@nestjs/common';
import { InjectRepository } from '@nestjs/typeorm';
import { Repository } from 'typeorm';
import { User } from './user.entity';
import * as bcrypt from 'bcryptjs';
@Injectable()
export class AuthService {
constructor(
@InjectRepository(User)
private usersRepository: Repository<User>,
) {}
async register(username: string, password: string): Promise<User> {
const hashedPassword = await bcrypt.hash(password, 10);
const user = this.usersRepository.create({
username,
password: hashedPassword,
});
return this.usersRepository.save(user);
}
async validateUser(username: string, password: string): Promise<User | null> {
const user = await this.usersRepository.findOne({ username });
if (!user) {
return null;
}
const isPasswordValid = await bcrypt.compare(password, user.password);
if (!isPasswordValid) {
return null;
}
return user;
}
}
```
最后,在您的控制器中,您可以使用 `@Body()` 装饰器获取来自 HTTP 请求的用户名和密码,并将其传递给 `AuthService` 服务的方法。例如:
```typescript
import { Controller, Post, Body } from '@nestjs/common';
import { AuthService } from './auth.service';
@Controller('auth')
export class AuthController {
constructor(private authService: AuthService) {}
@Post('register')
async register(
@Body('username') username: string,
@Body('password') password: string,
) {
const user = await this.authService.register(username, password);
return { id: user.id };
}
@Post('login')
async login(
@Body('username') username: string,
@Body('password') password: string,
) {
const user = await this.authService.validateUser(username, password);
if (!user) {
return { error: 'Invalid username or password' };
}
return { id: user.id };
}
}
```
以上是一个简单的 NestJS + TypeORM 注册用户和验证登录的实现。当用户注册时,它将哈希密码并将其保存到数据库中。在用户登录时,它将检索带有给定用户名的用户,并使用 bcrypt 将密码与存储的哈希值进行比较。如果它们匹配,则用户验证成功。
阅读全文
相关推荐



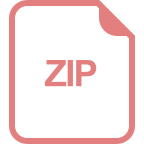
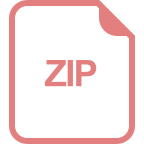
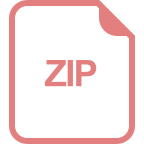
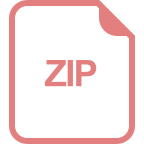
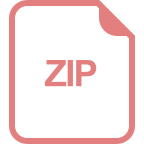
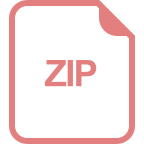
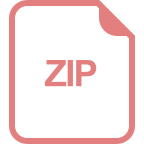
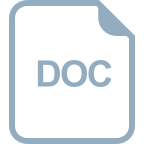




