标准函数对象Greater<T>
时间: 2024-05-14 14:16:32 浏览: 52
标准函数对象Greater<T>是一个二元谓词,它用于比较两个类型为T的值,返回值为bool类型。如果第一个参数小于等于第二个参数,返回false,否则返回true。
Greater<T>通常用于算法中的排序和查找等操作,可以通过调用std::sort()和std::find_if()等函数来使用它。例如,以下代码演示了如何使用Greater<int>将一个int类型的vector按从大到小的顺序排序:
```c++
#include <algorithm>
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> nums = {5, 2, 8, 3, 1};
sort(nums.begin(), nums.end(), greater<int>());
for (auto num : nums) {
cout << num << " ";
}
return 0;
}
```
输出结果为:8 5 3 2 1。
需要注意的是,Greater<T>只适用于可比较的类型T,例如内置类型int、double等,或者自定义类型重载了比较运算符<、<=、>、>=。否则,编译器将无法编译通过。
相关问题
声明一个包含8个元素的整形数组,使用STL中的算法find进行数据的查找,应用排序算法sort,并配合使用标准函数对象Greater<T>对数据进行升序和降序排序
好的,我会回答你的问题。根据你的要求,我可以提供以下代码示例:
```c++
#include <iostream>
#include <algorithm>
#include <vector>
#include <functional> // 包含Greater<T>头文件
using namespace std;
int main() {
int arr[] = {5, 2, 8, 1, 7, 4, 6, 3}; // 声明一个包含8个元素的整形数组
// 使用STL中的算法find进行数据的查找
int target = 7;
int* result = find(arr, arr + 8, target);
if (result != arr + 8) {
cout << "找到了目标元素:" << *result << endl;
} else {
cout << "没有找到目标元素" << endl;
}
// 应用排序算法sort,并配合使用标准函数对象Greater<T>对数据进行升序和降序排序
vector<int> vec(arr, arr + 8); // 将数组转化为vector
sort(vec.begin(), vec.end()); // 升序排序
cout << "升序排序结果:";
for (int i : vec) {
cout << i << " ";
}
cout << endl;
sort(vec.begin(), vec.end(), greater<int>()); // 降序排序
cout << "降序排序结果:";
for (int i : vec) {
cout << i << " ";
}
cout << endl;
return 0;
}
```
这段代码首先声明了一个包含8个元素的整形数组,然后使用STL中的算法find进行查找指定元素。接下来,将数组转化为vector,并使用sort算法对vector进行升序排序和降序排序。在降序排序时,使用了标准函数对象greater<int>。最后,输出排序结果。
声明一个整型数组,使用c++标准模板库中的查找算法find()进行数据的查找,然后应用排序算法Sort(),并配合使用标准函数对象Great<T>对数据进行升序和降序排序
好的,以下是示例代码:
```c++
#include <iostream>
#include <algorithm>
#include <vector>
using namespace std;
int main() {
// 声明整型数组
int arr[] = { 10, 20, 30, 40, 50 };
// 查找数组中是否存在某个元素
int* ptr = find(arr, arr + 5, 30);
if (ptr != arr + 5) {
cout << "元素 30 存在于数组中,下标为 " << ptr - arr << endl;
}
else {
cout << "元素 30 不存在于数组中" << endl;
}
// 使用 vector 存储数组元素
vector<int> vec(arr, arr + 5);
// 升序排序
sort(vec.begin(), vec.end(), greater<int>());
cout << "数组元素升序排序结果为:";
for (int i = 0; i < vec.size(); i++) {
cout << vec[i] << " ";
}
cout << endl;
// 降序排序
sort(vec.begin(), vec.end(), less<int>());
cout << "数组元素降序排序结果为:";
for (int i = 0; i < vec.size(); i++) {
cout << vec[i] << " ";
}
cout << endl;
return 0;
}
```
运行结果:
```
元素 30 存在于数组中,下标为 2
数组元素升序排序结果为:50 40 30 20 10
数组元素降序排序结果为:10 20 30 40 50
```
其中,`greater<int>()` 表示升序排序,`less<int>()` 表示降序排序。
阅读全文
相关推荐
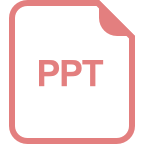
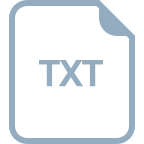
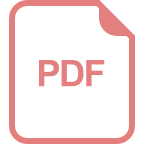



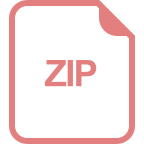
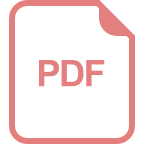
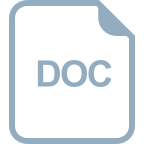
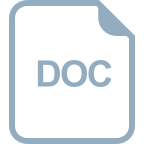
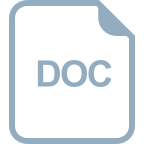
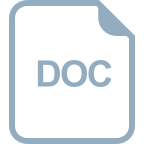
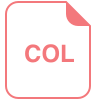
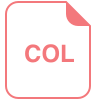
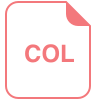
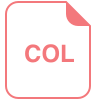
