在投票应用层协议项目的基础上,使用现代密码学算法,将该应用层协议修改为安全传输的通信过程。 方式一:将投票信息整体进行加解密; 方式二:将报文的某个字段进行加解密;两种方式分别描述,要求包含程序实现思路描述和关键代码的讲解
时间: 2024-03-23 13:43:19 浏览: 569
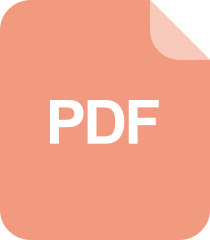
应用密码学:协议、算法与C源程序.中文版

好的,我会分别为你详细描述两种方式的实现。
方式一:将投票信息整体进行加解密
加密过程:
1. 客户端生成随机密钥K,并使用该密钥对投票信息进行AES对称加密。
```python
import base64
from Crypto.Cipher import AES
def encrypt_vote_info(vote_info, key):
# 将key转换为16字节的字符串
key = key.encode('utf-8')[:16]
# 将投票信息转换为字节流
vote_info = vote_info.encode('utf-8')
# 使用AES加密算法进行加密
cipher = AES.new(key, AES.MODE_ECB)
ciphertext = cipher.encrypt(vote_info)
# 将加密后的密文进行base64编码
ciphertext_base64 = base64.b64encode(ciphertext).decode('utf-8')
return ciphertext_base64
```
2. 客户端使用RSA公钥对随机密钥K进行非对称加密,并将加密后的密钥K'发送给服务器。
```python
from Crypto.PublicKey import RSA
def encrypt_key(key, public_key):
# 使用RSA公钥进行加密
cipher = PKCS1_v1_5.new(public_key)
ciphertext = cipher.encrypt(key.encode('utf-8'))
# 将加密后的密文进行base64编码
ciphertext_base64 = base64.b64encode(ciphertext).decode('utf-8')
return ciphertext_base64
```
解密过程:
1. 服务器使用RSA私钥对收到的密钥K'进行非对称解密,得到随机密钥K。
```python
from Crypto.Cipher import PKCS1_v1_5
def decrypt_key(ciphertext, private_key):
# 将密文进行base64解码
ciphertext = base64.b64decode(ciphertext.encode('utf-8'))
# 使用RSA私钥进行解密
cipher = PKCS1_v1_5.new(private_key)
key = cipher.decrypt(ciphertext, None).decode('utf-8')
return key
```
2. 服务器使用随机密钥K对收到的投票信息进行AES对称解密,得到原始的投票信息。
```python
def decrypt_vote_info(ciphertext, key):
# 将key转换为16字节的字符串
key = key.encode('utf-8')[:16]
# 将密文进行base64解码
ciphertext = base64.b64decode(ciphertext.encode('utf-8'))
# 使用AES加密算法进行解密
cipher = AES.new(key, AES.MODE_ECB)
vote_info = cipher.decrypt(ciphertext).decode('utf-8')
return vote_info
```
方式二:将报文的某个字段进行加解密
加密过程:
1. 客户端生成随机密钥K,并使用该密钥对需要加密的字段进行AES对称加密。
```python
import base64
from Crypto.Cipher import AES
def encrypt_field(field, key):
# 将key转换为16字节的字符串
key = key.encode('utf-8')[:16]
# 将字段转换为字节流
field = field.encode('utf-8')
# 使用AES加密算法进行加密
cipher = AES.new(key, AES.MODE_ECB)
ciphertext = cipher.encrypt(field)
# 将加密后的密文进行base64编码
ciphertext_base64 = base64.b64encode(ciphertext).decode('utf-8')
return ciphertext_base64
```
2. 客户端使用RSA公钥对随机密钥K进行非对称加密,并将加密后的密钥K'替换原始报文中的对应字段,得到加密后的报文。
```python
from Crypto.PublicKey import RSA
def encrypt_key(key, public_key, vote_msg):
# 使用RSA公钥进行加密
cipher = PKCS1_v1_5.new(public_key)
ciphertext = cipher.encrypt(key.encode('utf-8'))
# 将加密后的密文进行base64编码
ciphertext_base64 = base64.b64encode(ciphertext).decode('utf-8')
# 将加密后的密钥K'替换原始报文中的对应字段
vote_msg['key'] = ciphertext_base64
return vote_msg
```
解密过程:
1. 服务器使用RSA私钥对收到的密钥K'进行非对称解密,得到随机密钥K。
```python
from Crypto.Cipher import PKCS1_v1_5
def decrypt_key(ciphertext, private_key):
# 将密文进行base64解码
ciphertext = base64.b64decode(ciphertext.encode('utf-8'))
# 使用RSA私钥进行解密
cipher = PKCS1_v1_5.new(private_key)
key = cipher.decrypt(ciphertext, None).decode('utf-8')
return key
```
2. 服务器使用随机密钥K对收到的加密字段进行AES对称解密,得到原始的字段。
```python
def decrypt_field(ciphertext, key):
# 将key转换为16字节的字符串
key = key.encode('utf-8')[:16]
# 将密文进行base64解码
ciphertext = base64.b64decode(ciphertext.encode('utf-8'))
# 使用AES加密算法进行解密
cipher = AES.new(key, AES.MODE_ECB)
field = cipher.decrypt(ciphertext).decode('utf-8')
return field
```
以上是两种方式的实现思路和关键代码讲解,希望能对你有所帮助!
阅读全文
相关推荐
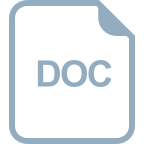
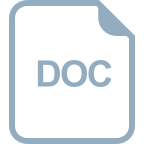



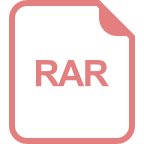
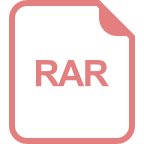
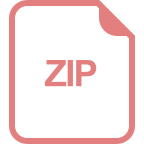
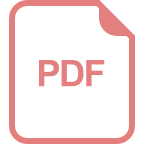
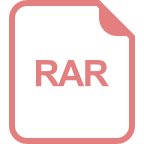
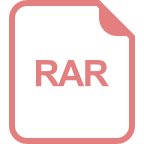
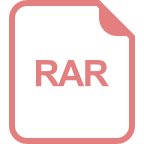
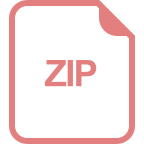
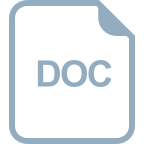
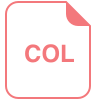
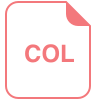
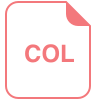
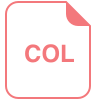