用qt写一个tcp服务器与客户端之间如何计算延时率的示例
时间: 2024-02-24 09:00:21 浏览: 23
以下是一个基于Qt的TCP服务器和客户端的延迟率示例:
服务器端代码:
```cpp
#include <QTcpServer>
#include <QTcpSocket>
#include <QDateTime>
class Server : public QTcpServer
{
Q_OBJECT
public:
explicit Server(QObject *parent = nullptr) : QTcpServer(parent)
{
m_socket = nullptr;
}
void start()
{
if (listen(QHostAddress::Any, 1234))
{
qDebug() << "Server started";
}
else
{
qDebug() << "Server could not start";
}
}
signals:
void latencyUpdated(qint64);
protected:
void incomingConnection(qintptr handle) override
{
if (m_socket != nullptr)
{
// Only allow one connection at a time
qWarning() << "Rejecting incoming connection";
QTcpSocket socket;
socket.setSocketDescriptor(handle);
socket.write("Already connected\n");
socket.flush();
socket.waitForBytesWritten();
socket.disconnectFromHost();
return;
}
m_socket = new QTcpSocket(this);
m_socket->setSocketDescriptor(handle);
connect(m_socket, &QTcpSocket::disconnected, this, [this]() {
qDebug() << "Client disconnected";
m_socket->deleteLater();
m_socket = nullptr;
});
connect(m_socket, &QTcpSocket::readyRead, this, [this]() {
qint64 now = QDateTime::currentMSecsSinceEpoch();
qint64 ping;
m_socket->read((char *)&ping, sizeof(ping));
ping = now - ping;
emit latencyUpdated(ping);
});
qDebug() << "Client connected";
}
private:
QTcpSocket *m_socket;
};
```
客户端代码:
```cpp
#include <QTcpSocket>
#include <QTimer>
#include <QDateTime>
class Client : public QObject
{
Q_OBJECT
public:
explicit Client(QObject *parent = nullptr) : QObject(parent)
{
m_socket = new QTcpSocket(this);
m_pingTimer = new QTimer(this);
connect(m_socket, &QTcpSocket::connected, this, [this]() {
qDebug() << "Connected to server";
connect(m_pingTimer, &QTimer::timeout, this, &Client::ping);
m_pingTimer->start(1000);
});
connect(m_socket, &QTcpSocket::disconnected, this, [this]() {
m_pingTimer->stop();
qDebug() << "Disconnected from server";
});
connect(m_socket, static_cast<void (QTcpSocket::*)(QAbstractSocket::SocketError)>(&QTcpSocket::error), this, [this](QAbstractSocket::SocketError socketError) {
m_pingTimer->stop();
qDebug() << "Error: " << socketError;
});
connect(m_socket, &QTcpSocket::readyRead, this, [this]() {
qint64 now = QDateTime::currentMSecsSinceEpoch();
qint64 pong;
m_socket->read((char *)&pong, sizeof(pong));
pong = now - pong;
qDebug() << "Latency: " << pong << "ms";
});
}
void connectToServer()
{
m_socket->connectToHost("localhost", 1234);
}
private slots:
void ping()
{
qint64 now = QDateTime::currentMSecsSinceEpoch();
m_socket->write((char *)&now, sizeof(now));
m_socket->flush();
}
private:
QTcpSocket *m_socket;
QTimer *m_pingTimer;
};
```
使用方法:
在主函数中创建一个服务器和一个客户端对象,并将客户端连接到服务器:
```cpp
Server server;
Client client;
QObject::connect(&server, &Server::latencyUpdated, &client, &Client::latencyUpdated);
server.start();
client.connectToServer();
```
这将启动一个服务器,监听端口1234,并将客户端连接到该端口。每秒钟,客户端会向服务器发送一个ping消息,服务器会在接收到该消息后记录当前时间,并将其发送回客户端作为pong消息。客户端接收到pong消息时,记录当前时间并计算延迟时间。服务器在接收到ping消息时记录当前时间并将其发送回客户端作为pong消息,客户端接收到pong消息时记录当前时间并计算延迟时间。由于网络延迟的不确定性,建议多次测量并计算平均值以获得更准确的延迟率。
相关推荐
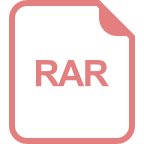
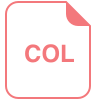
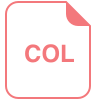
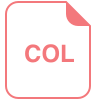











