写一段判断字符集是否是前缀码的C++代码
时间: 2024-04-09 10:29:16 浏览: 80
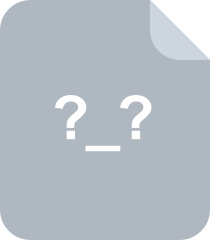
数据结构题前缀码判定.cpp

下面是一个简单的C++代码示例,用于判断给定字符集是否是前缀码:
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
struct Node {
char data;
bool isEndOfWord;
std::vector<Node*> children;
Node(char c) : data(c), isEndOfWord(false) {}
~Node() {
for (auto child : children) {
delete child;
}
}
};
class Trie {
private:
Node* root;
public:
Trie() : root(new Node('\0')) {}
void insert(const std::string& word) {
Node* current = root;
for (char c : word) {
Node* child = nullptr;
for (auto node : current->children) {
if (node->data == c) {
child = node;
break;
}
}
if (child == nullptr) {
child = new Node(c);
current->children.push_back(child);
}
current = child;
}
current->isEndOfWord = true;
}
bool isPrefixCode() const {
return isPrefixCode(root, "");
}
private:
bool isPrefixCode(Node* node, const std::string& prefix) const {
if (node == nullptr)
return true;
if (node->isEndOfWord && !node->children.empty()) {
return false; // 当前节点是某个单词的结束节点并且还有孩子节点,不是前缀码
}
for (Node* child : node->children) {
std::string newPrefix = prefix + child->data;
if (!isPrefixCode(child, newPrefix)) {
return false; // 子节点不是前缀码
}
}
return true;
}
};
int main() {
Trie trie;
int n;
std::cout << "请输入字符集的大小: ";
std::cin >> n;
std::cout << "请输入字符集: ";
for (int i = 0; i < n; i++) {
std::string word;
std::cin >> word;
trie.insert(word);
}
if (trie.isPrefixCode()) {
std::cout << "给定字符集是前缀码" << std::endl;
} else {
std::cout << "给定字符集不是前缀码" << std::endl;
}
return 0;
}
```
这个代码使用了前缀树(Trie)数据结构来判断给定字符集是否是前缀码。用户需要首先输入字符集的大小,然后逐个输入字符集中的字符串。程序会将这些字符串插入到前缀树中,并最后判断整个字符集是否构成一个前缀码。希望对你有所帮助!
阅读全文
相关推荐
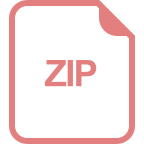
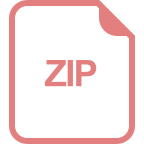
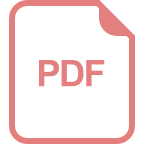
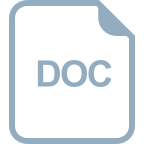
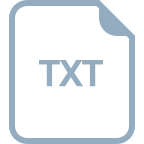
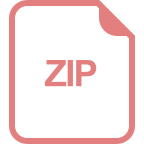
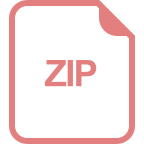
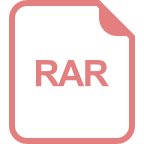
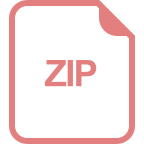
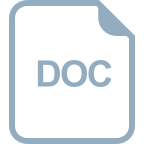
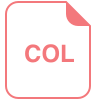
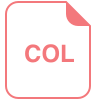
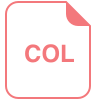
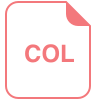
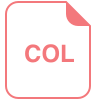