使用c++编写一个检查字符串value是否为空字符串的代码
时间: 2023-06-03 11:01:36 浏览: 80
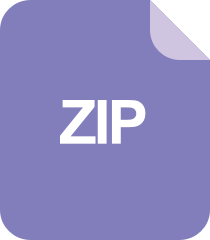
判断字符串是否是空
下面是使用C语言编写一个检查字符串是否为空字符串的代码:
```c
#include <stdio.h>
#include <string.h>
int main() {
char value[] = "Hello, World!";
if (strlen(value) == 0) {
printf("Value is an empty string\n");
} else {
printf("Value is not an empty string\n");
}
return 0;
}
```
首先,我们声明一个名为`value`的字符串,并将其赋值为`"Hello, World!"`。然后,使用`strlen()`函数检查`value`字符串的长度是否为0。如果长度为0,则表示`value`是一个空字符串;否则,`value`不是一个空字符串。最后,我们使用`printf()`函数输出相应的消息。
阅读全文
相关推荐
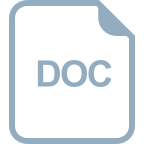
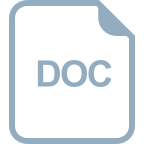













