java将多个对象保存到文件中
时间: 2024-04-29 18:21:40 浏览: 112
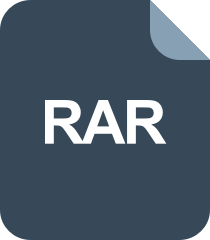
将多个文件保存到一个文件内
Java可以使用对象序列化来将多个对象保存到文件中。对象序列化是将一个对象转换为字节序列的过程,以便在需要时将其恢复到原始状态。以下是一些示例代码,演示如何将多个对象保存到文件中:
1. 创建一个类来表示要保存的对象,这个类必须实现Serializable接口。
```java
import java.io.Serializable;
public class Person implements Serializable {
private static final long serialVersionUID = 1L;
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
// getters and setters
}
```
2. 在主程序中创建一个ArrayList来存储要保存的对象。
```java
import java.util.ArrayList;
import java.io.*;
public class Main {
public static void main(String[] args) {
ArrayList<Person> people = new ArrayList<Person>();
people.add(new Person("Alice", 25));
people.add(new Person("Bob", 30));
people.add(new Person("Charlie", 35));
}
}
```
3. 使用ObjectOutputStream将ArrayList中的对象写入文件。
```java
import java.util.ArrayList;
import java.io.*;
public class Main {
public static void main(String[] args) {
ArrayList<Person> people = new ArrayList<Person>();
people.add(new Person("Alice", 25));
people.add(new Person("Bob", 30));
people.add(new Person("Charlie", 35));
try {
FileOutputStream fos = new FileOutputStream("people.ser");
ObjectOutputStream oos = new ObjectOutputStream(fos);
oos.writeObject(people);
oos.close();
fos.close();
System.out.println("Objects saved to file.");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
4. 使用ObjectInputStream从文件中读取对象并将它们放回ArrayList中。
```java
import java.util.ArrayList;
import java.io.*;
public class Main {
public static void main(String[] args) {
ArrayList<Person> people = null;
try {
FileInputStream fis = new FileInputStream("people.ser");
ObjectInputStream ois = new ObjectInputStream(fis);
people = (ArrayList<Person>) ois.readObject();
ois.close();
fis.close();
System.out.println("Objects loaded from file.");
} catch (IOException | ClassNotFoundException e) {
e.printStackTrace();
}
// do something with the ArrayList
for (Person p : people) {
System.out.println(p.getName() + " is " + p.getAge() + " years old.");
}
}
}
```
注意:在使用对象序列化时,类必须实现Serializable接口。此外,序列化的对象必须是可传输的,即它们的所有成员变量都必须是可序列化的。如果对象中有不可序列化的成员变量,那么在序列化时将会抛出NotSerializableException。
阅读全文
相关推荐
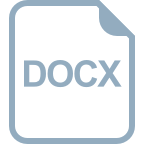
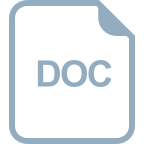
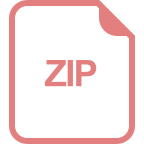
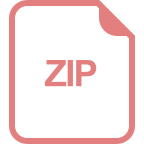
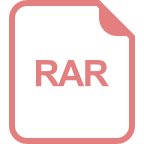
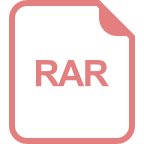
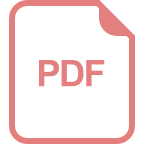
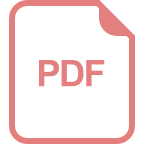
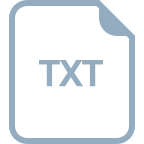
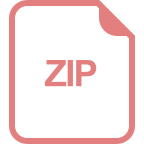
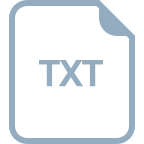
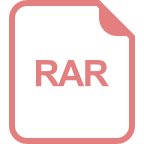
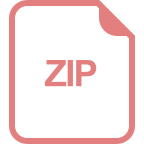
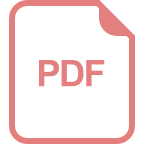
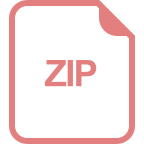
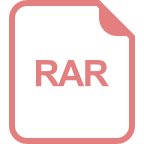
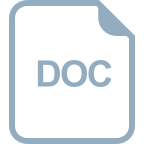