使用简化的类层次结构来表示公司目前和以前的雇员状况 c++
时间: 2023-07-14 22:13:26 浏览: 83
以下是使用简化的类层次结构来表示公司目前和以前的雇员状况的 c++ 代码示例:
```c++
#include <iostream>
using namespace std;
class Employee {
protected:
string name;
int age;
double salary;
public:
Employee(string n, int a, double s) : name(n), age(a), salary(s) {}
virtual void display() {
cout << "Name: " << name << endl;
cout << "Age: " << age << endl;
cout << "Salary: " << salary << endl;
}
};
class CurrentEmployee : public Employee {
private:
string department;
public:
CurrentEmployee(string n, int a, double s, string d) : Employee(n, a, s), department(d) {}
void display() {
Employee::display();
cout << "Department: " << department << endl;
}
};
class FormerEmployee : public Employee {
private:
string reasonForLeaving;
public:
FormerEmployee(string n, int a, double s, string r) : Employee(n, a, s), reasonForLeaving(r) {}
void display() {
Employee::display();
cout << "Reason for leaving: " << reasonForLeaving << endl;
}
};
int main() {
Employee* e1 = new CurrentEmployee("John", 30, 5000, "Sales");
Employee* e2 = new FormerEmployee("Jane", 25, 4000, "Moved to another city");
e1->display();
cout << endl;
e2->display();
delete e1;
delete e2;
return 0;
}
```
在这个例子中,我们有三个类:`Employee`,`CurrentEmployee`和`FormerEmployee`。`Employee`类是一个基类,它有一些共同的属性,如姓名、年龄和薪水,并且有一个虚函数`display()`来显示这些属性。`CurrentEmployee`和`FormerEmployee`都是从`Employee`类派生出来的类,它们分别表示当前雇员和以前的雇员,并具有特定于每个类型的属性。这些类都有一个`display()`函数来覆盖`Employee`类中的虚函数,以便添加新的属性并显示它们。在主函数中,我们创建了两个指向不同类型雇员对象的指针,并调用它们的`display()`函数来显示它们的属性。最后,我们释放了内存以避免内存泄漏。
相关推荐


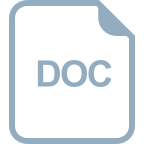
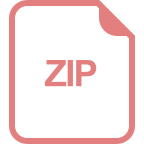
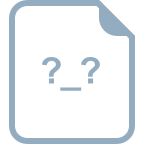
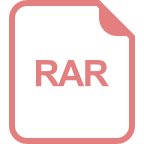
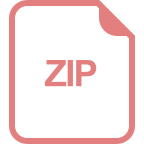
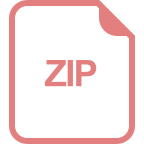
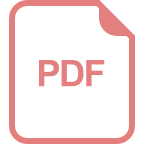
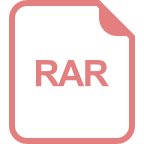
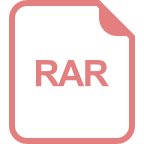
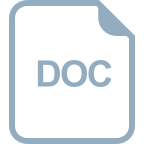
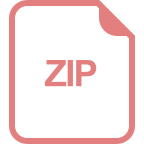
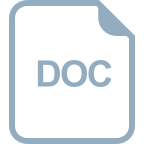
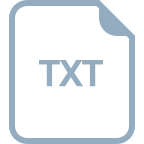