springboot配置swagger2
时间: 2023-05-31 08:19:38 浏览: 135
### 回答1:
要在Spring Boot应用程序中配置Swagger2,您可以按照以下步骤进行操作:
1.添加Swagger2依赖项:在pom.xml文件中,添加以下依赖项:
```
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
<version>2.9.2</version>
</dependency>
```
2.添加Swagger2配置类:创建一个Swagger2配置类,该类应该包含@Configuration和@EnableSwagger2注释,并提供有关API文档的信息,例如标题,描述和版本号。
```
@Configuration
@EnableSwagger2
public class SwaggerConfig {
@Bean
public Docket api() {
return new Docket(DocumentationType.SWAGGER_2)
.select()
.apis(RequestHandlerSelectors.any())
.paths(PathSelectors.any())
.build()
.apiInfo(apiInfo());
}
private ApiInfo apiInfo() {
return new ApiInfoBuilder()
.title("My API")
.description("API for My Application")
.version("1.0")
.build();
}
}
```
3.启用Swagger2 UI:在您的应用程序类中,添加@EnableSwagger2注释,以便启用Swagger2 UI。
```
@SpringBootApplication
@EnableSwagger2
public class MyApp {
public static void main(String[] args) {
SpringApplication.run(MyApp.class, args);
}
}
```
4.测试API文档:运行应用程序并访问http://localhost:8080/swagger-ui.html,您将看到Swagger2 UI,其中包含您的API文档的详细信息。
希望这可以帮助您配置Spring Boot中的Swagger2。
### 回答2:
Swagger2是现今广泛使用的RESTful API文档自动生成工具,它可以帮助我们生成美观易懂的API文档,并提供了一系列交互式的API测试工具。Spring Boot是一款开箱即用的轻量级框架,能够帮助您快速搭建Java Web应用程序。本篇文章将介绍如何在Spring Boot项目中集成Swagger2来生成API文档,以及如何快速测试我们的API。
第一步: 添加相应依赖
首先,在您的Spring Boot项目的pom文件中添加以下依赖:
```xml
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
<version>2.9.2</version>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger-ui</artifactId>
<version>2.9.2</version>
</dependency>
```
第二步: 配置Swagger2
接下来,在您的Spring Boot项目的配置类中添加以下代码:
```java
@Configuration
@EnableSwagger2
public class SwaggerConfig {
@Bean
public Docket api() {
return new Docket(DocumentationType.SWAGGER_2)
.select()
.apis(RequestHandlerSelectors.basePackage("<your package name>"))
.paths(PathSelectors.any())
.build()
.apiInfo(apiInfo());
}
private ApiInfo apiInfo() {
return new ApiInfoBuilder()
.title("<Your API Title>")
.version("<Your API version>")
.description("<Your API description>")
.termsOfServiceUrl("<Your terms of service URL>")
.contact("<Your contact details>")
.license("<Your license>")
.licenseUrl("<Your license URL>")
.build();
}
}
```
请注意,您需要在“<your package name>”处提供包名,该包名包含您要暴露为RESTful API的类。 此外,您还需要设置API的基本信息,例如名称,版本,描述等。
第三步: 可选配置
现在您已经成功添加了Swagger2依赖项并配置了Swagger2的基本信息,现在您可以添加一些可选配置来改进生成的API文档。例如,您可以通过以下方法来解决API文档中显示“/error”的问题:
```java
@Configuration
@EnableSwagger2
public class SwaggerConfig {
// ...
@Bean
public UiConfiguration uiConfiguration() {
return UiConfigurationBuilder.builder().displayRequestDuration(true).build();
}
@Bean
public ErrorModelBuilder errorModelBuilder() {
return new DefaultErrorModelBuilder();
}
}
```
这将生成更好的API文档。
第四步:快速测试API
已经成功地将Swagger2集成到Spring Boot项目中!现在可以使用Swagger界面,以交互方式测试您的API。
在“http://localhost:8080/swagger-ui.html”上启动项目,以查看自动生成的API文档页面。
自动生成的UI界面上有完整的API信息,UI有7大模块:
- Swagger UI界面展示了 API 文档中的所有接口。
- Model展示了定义的模型。
- Model Schema是对每个模型对象的结构定义,其包含模型对象的属性、类型以及描述信息。
- Parameters显示模型中的所有模板参数。
- Response Messages显示所有返回的消息。
- Authorizations中定义了API的访问需要的身份验证信息。
- 外部文档文档页面可以添加外部文档或链接以供使用者查看。
您可以点击任何API并使用交互式工具进行测试。 例如,您可以点击“/users”接口,然后使用swagger UI的“Try it out”按钮,输入查询参数,然后点击“Execute”进行测试。您还可以通过在请求正文中指定JSON或XML等有效负载,并将其发送到API来测试其余功能。自动测试将显示返回的响应,并显示响应的HTTP状态代码。
### 回答3:
SpringBoot是一个开源的Java框架,可以快速搭建Web应用程序。Swagger2是一个开源的API框架,可以帮助Java开发人员快速构建RESTful Web服务,并生成API文档。SpringBoot与Swagger2相结合,可以大大简化Web应用程序的开发过程,并生成易于使用和阅读的API文档。
一、 pom.xml文件添加Swagger2依赖
在pom.xml文件中添加Swagger2依赖:
```xml
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
<version>2.9.2</version>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger-ui</artifactId>
<version>2.9.2</version>
</dependency>
```
二、 配置Swagger2
在SpringBoot的主应用程序类中添加Swagger2的配置信息:
```java
@Configuration
@EnableSwagger2
public class SwaggerConfig {
@Bean
public Docket createRestApi() {
return new Docket(DocumentationType.SWAGGER_2)
.apiInfo(apiInfo())
.select()
.apis(RequestHandlerSelectors.basePackage("com.example.demo.controller"))
.paths(PathSelectors.any())
.build();
}
private ApiInfo apiInfo() {
return new ApiInfoBuilder()
.title("SpringBoot集成Swagger2")
.description("详细信息......")
.version("1.0.0")
.build();
}
}
```
其中,@Configuration注解告诉SpringBoot这是一个配置文件;@EnableSwagger2注解启用Swagger2;createRestApi()方法返回一个Docket类实例,这个类用来配置Swagger2的基本信息;apiInfo()方法用来配置API文档的基本信息。
三、 使用Swagger2
启动SpringBoot应用程序,访问http://localhost:端口号/swagger-ui.html,就可以看到自动生成的API文档了。
总结:
SpringBoot与Swagger2相结合,可以大大简化Web应用程序的开发过程,并生成易于使用和阅读的API文档。其中,Swagger2的配置非常简单,只需要在pom.xml文件中添加Swagger2依赖,并在SpringBoot应用程序的主应用程序类中添加Swagger2的配置信息即可。
相关推荐
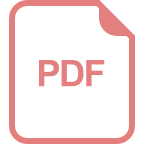
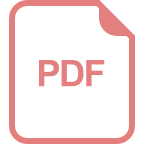














