帮我用c语言链表写一个可以实现多行输入的KWIC索引程序
时间: 2023-04-07 14:03:28 浏览: 140
好的,我可以回答这个问题。以下是一个基于链表的C语言KWIC索引程序的示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LINE_LEN 1000
#define MAX_WORD_LEN 100
typedef struct Node {
char *line;
struct Node *next;
} Node;
void insert(Node **head, char *line) {
Node *new_node = (Node *) malloc(sizeof(Node));
new_node->line = line;
new_node->next = NULL;
if (*head == NULL) {
*head = new_node;
} else {
Node *curr = *head;
while (curr->next != NULL) {
curr = curr->next;
}
curr->next = new_node;
}
}
void print_kwic(Node *head) {
Node *curr = head;
while (curr != NULL) {
char *line = curr->line;
int len = strlen(line);
char *words[MAX_WORD_LEN];
int word_count = 0;
// Split line into words
char *word = strtok(line, " ");
while (word != NULL) {
words[word_count++] = word;
word = strtok(NULL, " ");
}
// Print all rotations of the line
for (int i = 0; i < word_count; i++) {
for (int j = 0; j < word_count; j++) {
printf("%s ", words[(i + j) % word_count]);
}
printf("\n");
}
curr = curr->next;
}
}
int main() {
Node *head = NULL;
char line[MAX_LINE_LEN];
// Read input lines
while (fgets(line, MAX_LINE_LEN, stdin) != NULL) {
int len = strlen(line);
if (line[len - 1] == '\n') {
line[len - 1] = '\0';
}
insert(&head, strdup(line));
}
// Print KWIC index
print_kwic(head);
// Free memory
Node *curr = head;
while (curr != NULL) {
Node *next = curr->next;
free(curr->line);
free(curr);
curr = next;
}
return 0;
}
```
这个程序可以从标准输入中读取多行输入,并输出它们的KWIC索引。KWIC索引是一种文本索引方法,它将每行文本旋转成所有可能的位置,并按字母顺序排序,以便更容易地查找特定单词。
请注意,这个程序并不完美,可能存在一些边界情况和错误处理问题,但它可以作为一个基本的参考实现。
阅读全文
相关推荐




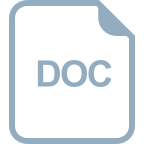

