Java springboot中如何设计并实现将全局异常记录在数据库中进行管理
时间: 2024-03-21 10:40:29 浏览: 18
在 Spring Boot 中,可以使用 Spring AOP 和 Spring Data JPA 技术来实现全局异常记录在数据库中进行管理。具体实现步骤如下:
1. 定义一个异常日志实体类,包含异常信息、调用方法、调用参数、请求 IP、异常时间等信息。
2. 定义一个全局异常处理切面类,使用 `@Aspect` 注解和 `@Around` 注解实现对所有方法的异常处理。在异常处理方法中,将异常信息封装到异常日志实体类中,并调用 Repository 层将日志信息保存到数据库中。
3. 在 Spring 配置文件中配置 AOP 切面和数据源等信息。
下面是一个简单的示例代码:
异常日志实体类:
```
@Entity
@Table(name = "exception_log")
public class ExceptionLog {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String exceptionMessage;
private String methodName;
private String methodArgs;
private String requestIp;
private Date createTime;
// getters and setters
}
```
异常日志 Repository 接口:
```
@Repository
public interface ExceptionLogRepository extends JpaRepository<ExceptionLog, Long> {
}
```
全局异常处理切面类:
```
@Aspect
@Component
public class ExceptionLogAspect {
@Autowired
private ExceptionLogRepository exceptionLogRepository;
@Around("execution(* com.example..*.*(..))")
public Object handleException(ProceedingJoinPoint joinPoint) throws Throwable {
Object result;
try {
result = joinPoint.proceed();
} catch (Exception e) {
ExceptionLog exceptionLog = new ExceptionLog();
exceptionLog.setExceptionMessage(e.getMessage());
exceptionLog.setMethodName(joinPoint.getSignature().toShortString());
exceptionLog.setMethodArgs(Arrays.toString(joinPoint.getArgs()));
exceptionLog.setRequestIp(getRequestIp());
exceptionLog.setCreateTime(new Date());
exceptionLogRepository.save(exceptionLog);
throw e;
}
return result;
}
private String getRequestIp() {
// get request ip
}
}
```
在 Spring 配置文件中配置 AOP 切面和数据源等信息:
```
@Configuration
@EnableAspectJAutoProxy
@EnableJpaRepositories(basePackages = "com.example.repository")
@EntityScan(basePackages = "com.example.entity")
public class AppConfig {
@Bean
public ExceptionLogAspect exceptionLogAspect() {
return new ExceptionLogAspect();
}
}
```
这样,在应用程序中发生异常时,就会将异常信息保存到数据库中,方便管理和排查问题。
相关推荐
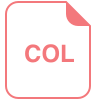
















