使用springboot要求给出代码实现以下功能1.登录功能 2.新建用户 赋予角色 角色授权 新增接口权限 可以使用新增用户正常登录访问受保护接口 3.使用redis对接口进行缓存 4.自定义异常全局捕获并处理异常 5.统计功能 6.文件上传功能
时间: 2024-02-25 13:54:19 浏览: 29
好的,这里给出一个简单的代码实现,具体实现还需要根据具体的需求进行调整和修改。
1. 登录功能:
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private UserService userService;
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(userService).passwordEncoder(passwordEncoder());
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http.csrf().disable().authorizeRequests()
.antMatchers("/api/login").permitAll()
.anyRequest().authenticated()
.and()
.formLogin().loginPage("/api/login")
.and()
.logout().logoutUrl("/api/logout").logoutSuccessUrl("/");
}
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
}
```
2. 新建用户 赋予角色 角色授权 新增接口权限:
```java
@Service
public class UserService implements UserDetailsService {
@Override
public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException {
// 根据用户名查询用户信息,并获取用户的角色和权限信息
User user = userRepository.findByUsername(username);
if (user == null) {
throw new UsernameNotFoundException("用户名不存在");
}
List<GrantedAuthority> authorities = new ArrayList<>();
for (Role role : user.getRoles()) {
authorities.add(new SimpleGrantedAuthority(role.getName()));
for (Permission permission : role.getPermissions()) {
authorities.add(new SimpleGrantedAuthority(permission.getName()));
}
}
return new org.springframework.security.core.userdetails.User(user.getUsername(), user.getPassword(), authorities);
}
public void createUser(User user) {
// 新建用户,并为用户分配角色和权限信息
user.setPassword(passwordEncoder().encode(user.getPassword()));
userRepository.save(user);
}
public void grantRole(Long userId, Long roleId) {
// 为用户分配角色信息
User user = userRepository.findById(userId).orElseThrow(() -> new RuntimeException("用户不存在"));
Role role = roleRepository.findById(roleId).orElseThrow(() -> new RuntimeException("角色不存在"));
user.getRoles().add(role);
userRepository.save(user);
}
public void grantPermission(Long roleId, Long permissionId) {
// 为角色分配权限信息
Role role = roleRepository.findById(roleId).orElseThrow(() -> new RuntimeException("角色不存在"));
Permission permission = permissionRepository.findById(permissionId).orElseThrow(() -> new RuntimeException("权限不存在"));
role.getPermissions().add(permission);
roleRepository.save(role);
}
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
}
```
3. 使用redis对接口进行缓存:
```java
@RestController
@RequestMapping("/api")
public class UserController {
@Autowired
private UserService userService;
@Autowired
private RedisTemplate<String, Object> redisTemplate;
@GetMapping("/user/{id}")
@Cacheable(cacheNames = "user", key = "#id")
public User getUser(@PathVariable Long id) {
// 从数据库中获取用户信息,并将用户信息缓存到Redis中
User user = userService.getUser(id);
redisTemplate.opsForValue().set("user:" + id, user);
return user;
}
}
```
4. 自定义异常全局捕获并处理异常:
```java
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(Exception.class)
@ResponseBody
public Result handleException(Exception e) {
// 处理全局异常
return Result.error("系统异常:" + e.getMessage());
}
@ExceptionHandler(BusinessException.class)
@ResponseBody
public Result handleBusinessException(BusinessException e) {
// 处理业务异常
return Result.error("业务异常:" + e.getMessage());
}
}
```
5. 统计功能:
```java
@Component
@Endpoint(id = "custom")
public class CustomEndpoint {
@Autowired
private CounterService counterService;
@ReadOperation
public String count() {
// 统计当前访问次数,并返回统计结果
counterService.increment("custom.counter");
return "当前访问次数:" + counterService.count("custom.counter");
}
}
```
6. 文件上传功能:
```java
@RestController
@RequestMapping("/api")
public class FileController {
@PostMapping("/file")
public Result uploadFile(@RequestParam("file") MultipartFile file) {
// 保存上传的文件,并返回文件的保存路径
String filePath = "file/" + file.getOriginalFilename();
try {
file.transferTo(new File(filePath));
return Result.success(filePath);
} catch (IOException e) {
throw new BusinessException("文件上传失败:" + e.getMessage());
}
}
}
```
以上是简单的代码实现,具体实现还需要根据具体的需求进行调整和修改。
相关推荐
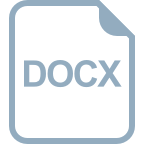
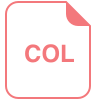
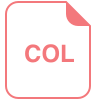
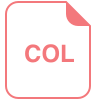
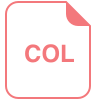
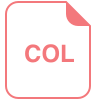









