用c++编写复数类的友元函数的双目运算符重载代码
时间: 2023-06-16 12:07:42 浏览: 62
下面是一个用 C++ 编写的复数类,包含了友元函数的双目运算符重载代码:
```cpp
#include<iostream>
using namespace std;
class Complex {
private:
double real;
double imag;
public:
Complex(double r = 0, double i = 0) {
real = r;
imag = i;
}
friend Complex operator+ (Complex const &, Complex const &);
friend Complex operator- (Complex const &, Complex const &);
friend Complex operator* (Complex const &, Complex const &);
friend Complex operator/ (Complex const &, Complex const &);
void print() {
cout << real << " + i" << imag << endl;
}
};
Complex operator+ (Complex const &c1, Complex const &c2) {
return Complex(c1.real + c2.real, c1.imag + c2.imag);
}
Complex operator- (Complex const &c1, Complex const &c2) {
return Complex(c1.real - c2.real, c1.imag - c2.imag);
}
Complex operator* (Complex const &c1, Complex const &c2) {
return Complex(c1.real*c2.real - c1.imag*c2.imag, c1.real*c2.imag + c1.imag*c2.real);
}
Complex operator/ (Complex const &c1, Complex const &c2) {
double r = c2.real*c2.real + c2.imag*c2.imag;
return Complex((c1.real*c2.real + c1.imag*c2.imag) / r, (c1.imag*c2.real - c1.real*c2.imag) / r);
}
int main() {
Complex c1(3, 4), c2(2, 3);
Complex c3 = c1 + c2;
Complex c4 = c1 - c2;
Complex c5 = c1 * c2;
Complex c6 = c1 / c2;
c3.print();
c4.print();
c5.print();
c6.print();
return 0;
}
```
在上面的代码中,我们使用了 `friend` 关键字来声明双目运算符重载函数为 `Complex` 类的友元函数,从而可以访问 `Complex` 类的私有成员变量 `real` 和 `imag`。同时,我们还重载了加、减、乘、除四种运算符,其中加、减、乘、除的运算结果均为复数。最后,我们在 `main` 函数中测试了这四种运算符的重载效果,输出了运算结果。
相关推荐
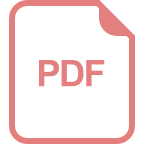
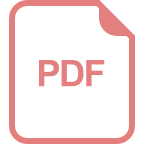














