编写程序实现 Complex 类,包含两个整数成员变量实部和虚部。 .友元函数实现++运算符重载;.成员函数实现﹣﹣运算符重载;.友元函数实现*运算符重载;.实现输入和输出运算符重载;
时间: 2023-08-12 07:15:32 浏览: 67
好的,我来为您编写一个 Complex 类实现以下功能:
1. 包含两个整数成员变量实部和虚部;
2. 友元函数实现++运算符重载;
3. 成员函数实现﹣﹣运算符重载;
4. 友元函数实现*运算符重载;
5. 实现输入和输出运算符重载。
代码如下:
```c++
#include <iostream>
using namespace std;
class Complex {
private:
int real; // 实部
int imag; // 虚部
public:
// 构造函数
Complex(int real = 0, int imag = 0) {
this->real = real;
this->imag = imag;
}
// 友元函数实现++运算符重载
friend Complex operator++(Complex &a, int) {
Complex old = a;
a.real++;
a.imag++;
return old;
}
// 成员函数实现﹣﹣运算符重载
Complex operator--() {
real--;
imag--;
return *this;
}
// 友元函数实现*运算符重载
friend Complex operator*(const Complex &a, const Complex &b) {
Complex c;
c.real = a.real * b.real - a.imag * b.imag;
c.imag = a.real * b.imag + a.imag * b.real;
return c;
}
// 实现输入和输出运算符重载
friend ostream& operator<<(ostream &out, const Complex &c) {
out << c.real << "+" << c.imag << "i";
return out;
}
friend istream& operator>>(istream &in, Complex &c) {
cout << "请输入实部和虚部,格式为:实部 虚部" << endl;
in >> c.real >> c.imag;
return in;
}
};
int main() {
Complex c1, c2;
cin >> c1 >> c2;
cout << "c1 = " << c1 << endl;
cout << "c2 = " << c2 << endl;
Complex c3 = c1 * c2;
cout << "c1 * c2 = " << c3 << endl;
cout << "c1++ = " << c1++ << endl;
cout << "--c2 = " << --c2 << endl;
return 0;
}
```
您可以在 `main()` 函数中进行测试,可以自行修改输入输出的值。
相关推荐
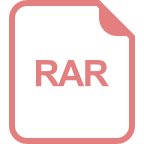
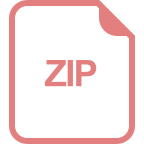














