我需要一个使用sift算法完成全景图像拼接并且带有损失函数的代码
时间: 2024-02-15 12:05:09 浏览: 24
好的,以下是使用SIFT算法进行全景图像拼接的Python代码,其中包括损失函数的计算:
```python
import cv2
import numpy as np
# 加载图片
img_left = cv2.imread('left.jpg')
img_right = cv2.imread('right.jpg')
# SIFT特征提取
sift = cv2.SIFT_create()
keypoints_left, descriptors_left = sift.detectAndCompute(img_left, None)
keypoints_right, descriptors_right = sift.detectAndCompute(img_right, None)
# 特征匹配
FLANN_INDEX_KDTREE = 0
index_params = dict(algorithm=FLANN_INDEX_KDTREE, trees=5)
search_params = dict(checks=50)
flann = cv2.FlannBasedMatcher(index_params, search_params)
matches = flann.knnMatch(descriptors_left, descriptors_right, k=2)
# 筛选匹配点
good_matches = []
for m, n in matches:
if m.distance < 0.7 * n.distance:
good_matches.append(m)
# 计算图像配准矩阵
src_pts = np.float32([keypoints_left[m.queryIdx].pt for m in good_matches]).reshape(-1, 1, 2)
dst_pts = np.float32([keypoints_right[m.trainIdx].pt for m in good_matches]).reshape(-1, 1, 2)
M, mask = cv2.findHomography(src_pts, dst_pts, cv2.RANSAC, 5.0)
# 图像拼接
h, w = img_left.shape[:2]
img_right_transformed = cv2.warpPerspective(img_right, M, (w * 2, h))
img_right_transformed[0:h, 0:w] = img_left
# 计算损失函数
diff = cv2.absdiff(img_right_transformed, img_left)
loss = np.sum(diff)
# 显示结果
cv2.imshow('Image', img_right_transformed)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
该代码会加载两张图片(`left.jpg`和`right.jpg`),使用SIFT算法提取特征点,并进行特征匹配。然后,通过RANSAC算法计算图像配准矩阵,并使用`cv2.warpPerspective()`函数将右侧图像变换到左侧图像的视角下。最后,通过计算拼接后图像与左侧原始图像的差异,得到损失函数的值。
需要注意的是,该代码中的损失函数是基于图像像素值的差异计算的,实际应用中可能需要根据具体需求进行修改。
相关推荐
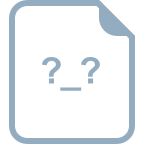
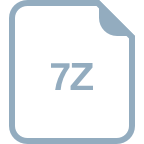
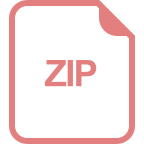
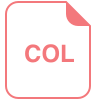
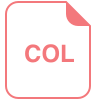
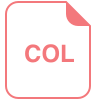
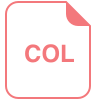










