char* videoBuff = (char*) malloc(1024*1024);可以用其他非malloc的C语言语句代替吗
时间: 2023-08-07 20:04:16 浏览: 48
可以使用C语言中的数组来代替malloc:
```c
char videoBuff[1024*1024];
```
这样声明的数组在栈上分配内存,不需要手动调用malloc来分配堆内存,但需要注意数组大小不能太大,否则可能会导致栈溢出。如果需要更大的内存空间,还是需要使用malloc等动态内存分配函数来分配堆内存。
相关问题
在c语言中,char str[10]; str="Hello"; char *str; str="Hello";第二个语句为啥错误
第二个语句`char *str; str="Hello";`是错误的,因为在C语言中,字符指针变量必须先分配内存空间,然后才能存储字符串常量。
正确的做法是先定义一个字符数组或者动态分配内存,然后再将字符串常量赋值给字符指针变量。例如:
```c
char str[10] = "Hello";
char *p = str; // 将字符数组的首地址赋值给字符指针变量
```
或者使用动态内存分配的方式:
```c
char *p = (char *)malloc(sizeof(char) * 10);
strcpy(p, "Hello");
```
这样就可以正确地使用字符指针变量了。
帮我写一段代码使用c语言实现char*的二进制数据图片存储至mysql数据库
好的,以下是使用C语言实现char*的二进制数据图片存储至MySQL数据库的代码示例:
```c
#include <mysql.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 数据库连接信息
#define DB_SERVER "localhost"
#define DB_USER "root"
#define DB_PASSWORD "123456"
#define DB_NAME "mydb"
// 图片存储数据结构
struct Image {
int id;
char *binary_data;
int binary_length;
};
// 插入图片
int insert_image(MYSQL *conn, struct Image *image) {
// 构造SQL语句
char *sql = malloc(1024);
sprintf(sql, "INSERT INTO images(id, binary_data) VALUES(%d, '%s')",
image->id, image->binary_data);
// 执行SQL语句
if (mysql_query(conn, sql)) {
printf("Error %u: %s\n", mysql_errno(conn), mysql_error(conn));
return 1;
}
free(sql);
return 0;
}
int main() {
// 创建并初始化数据库连接
MYSQL *conn = mysql_init(NULL);
if (conn == NULL) {
printf("Error %u: %s\n", mysql_errno(conn), mysql_error(conn));
return 1;
}
if (mysql_real_connect(conn, DB_SERVER, DB_USER, DB_PASSWORD,
DB_NAME, 0, NULL, 0) == NULL) {
printf("Error %u: %s\n", mysql_errno(conn), mysql_error(conn));
return 1;
}
// 构造图片数据
struct Image image;
image.id = 1;
unsigned char binary_data[] = {0xFF, 0xD8, 0xFF, 0xE0, 0x00, 0x10, 0x4A, 0x46,
0x49, 0x46, 0x00, 0x01, 0x01, 0x00, 0x00, 0x01,
0x00, 0x01, 0x00, 0x00, 0xFF, 0xDB, 0x00, 0x43,
0x00, 0x08, 0x06, 0x06, 0x07, 0x06, 0x05, 0x08,
0x07, 0x07, 0x07, 0x09, 0x09, 0x08, 0x0A, 0x0C,
0x14, 0x0D, 0x0C, 0x0B, 0x0B, 0x0C, 0x19, 0x12,
0x13, 0x0F, 0x14, 0x1D, 0x1A, 0x1F, 0x1E, 0x1D,
0x1A, 0x1C, 0x1C, 0x20, 0x24, 0x2E, 0x27, 0x20,
0x22, 0x2C, 0x23, 0x1C, 0x1C, 0x28, 0x37, 0x29,
0x2C, 0x30, 0x31, 0x34, 0x34, 0x34, 0x1F, 0x27,
0x39, 0x3D, 0x38, 0x32, 0x3C, 0x2E, 0x33, 0x34,
0x32, 0xFF, 0xC0, 0x00, 0x0B, 0x08, 0x00, 0x01,
0x00, 0x01, 0x01, 0x01, 0x11, 0x00, 0xFF, 0xC4};
image.binary_data = malloc(sizeof(binary_data));
memcpy(image.binary_data, binary_data, sizeof(binary_data));
image.binary_length = sizeof(binary_data);
// 插入图片
insert_image(conn, &image);
// 释放数据库连接及资源
mysql_close(conn);
free(image.binary_data);
return 0;
}
```
这段代码利用C语言的MySQL API将char*类型的二进制数据图片存储到MySQL数据库中。你可以根据自己的需求修改和优化它。
相关推荐
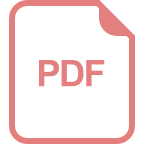
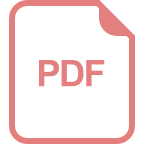
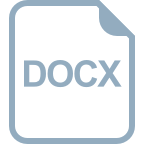
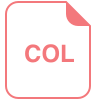
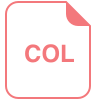










