使用Java实现测量身体肥瘦指数公式:BMI值(BMI=体重/(身高*身高) BMI在18-25之间为标准。体重单位为公斤,身高单位为米 。 为了防止用户输入错误,请编写自定义异常类,一个是身高值XX不正常,一个是体重值不正确。 (1)请用户输入身高和体重,进行下面的判断: 当用户输入的身高超过2.5或小于0.5时,调用异常显示"身高输入错误, xxx不是合理的身高 当用户输入的体重超过300或小于20时,调用异常显示"体重输入错误, xxx不是合理的体重 如果正确,就输出他的身体BMI并输出标准还是肥胖还是偏瘦。 (2)定义一个Person类,里面有身高和体重成员变量,有一个BMI()计算他的BMI值,并有一个show()方法输出他的BMI和身高和体重情况。
时间: 2024-02-24 21:59:42 浏览: 19
好的,我会根据你的要求进行回答。
首先,我们来定义自定义异常类HeightException和WeightException:
```java
class HeightException extends Exception {
public HeightException(String msg) {
super(msg);
}
}
class WeightException extends Exception {
public WeightException(String msg) {
super(msg);
}
}
```
然后,我们来实现测量身体肥瘦指数公式:
```java
import java.util.Scanner;
public class BMI {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.print("请输入身高(单位:米):");
double height = sc.nextDouble();
try {
if (height < 0.5 || height > 2.5) {
throw new HeightException("身高输入错误," + height + "不是合理的身高");
}
} catch (HeightException e) {
System.out.println(e.getMessage());
return;
}
System.out.print("请输入体重(单位:公斤):");
double weight = sc.nextDouble();
try {
if (weight < 20 || weight > 300) {
throw new WeightException("体重输入错误," + weight + "不是合理的体重");
}
} catch (WeightException e) {
System.out.println(e.getMessage());
return;
}
double bmi = weight / (height * height);
System.out.println("您的BMI值为:" + bmi);
if (bmi < 18) {
System.out.println("您的体型偏瘦");
} else if (bmi >= 18 && bmi <= 25) {
System.out.println("您的体型标准");
} else {
System.out.println("您的体型肥胖");
}
}
}
```
现在,我们来定义Person类:
```java
class Person {
private double height;
private double weight;
public Person(double height, double weight) {
this.height = height;
this.weight = weight;
}
public double getBMI() {
return weight / (height * height);
}
public void show() {
System.out.println("身高:" + height + "米");
System.out.println("体重:" + weight + "公斤");
System.out.println("BMI值:" + getBMI());
if (getBMI() < 18) {
System.out.println("体型偏瘦");
} else if (getBMI() >= 18 && getBMI() <= 25) {
System.out.println("体型标准");
} else {
System.out.println("体型肥胖");
}
}
}
```
然后,我们可以在main方法中实例化Person对象,调用show()方法来输出身高、体重、BMI值以及体型情况:
```java
public class Test {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.print("请输入身高(单位:米):");
double height = sc.nextDouble();
try {
if (height < 0.5 || height > 2.5) {
throw new HeightException("身高输入错误," + height + "不是合理的身高");
}
} catch (HeightException e) {
System.out.println(e.getMessage());
return;
}
System.out.print("请输入体重(单位:公斤):");
double weight = sc.nextDouble();
try {
if (weight < 20 || weight > 300) {
throw new WeightException("体重输入错误," + weight + "不是合理的体重");
}
} catch (WeightException e) {
System.out.println(e.getMessage());
return;
}
Person p = new Person(height, weight);
p.show();
}
}
```
相关推荐
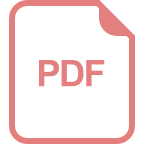
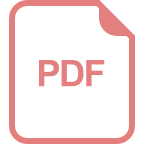







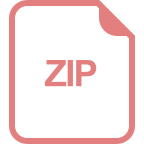
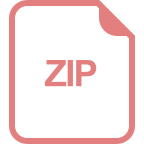
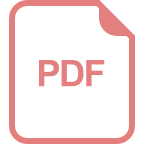
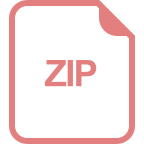
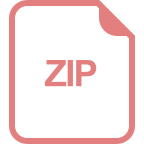