基于springboot设计能接入 http mqtt coap协议,并能把接受到的数据JSON格式化的物联网平台,写出示范代码,包含依赖项,import语句
时间: 2023-11-27 09:02:27 浏览: 56
以下是一个基于Spring Boot设计的可以接入http, mqtt, coap协议的物联网平台示范代码,包含依赖项和import语句:
依赖项:
```xml
<dependencies>
<!-- Spring Boot Web Starter -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- Eclipse Paho - MQTT Client -->
<dependency>
<groupId>org.eclipse.paho</groupId>
<artifactId>org.eclipse.paho.client.mqttv3</artifactId>
<version>1.2.5</version>
</dependency>
<!-- Californium - CoAP Framework -->
<dependency>
<groupId>org.eclipse.californium</groupId>
<artifactId>californium-core</artifactId>
<version>2.0.0-M7</version>
</dependency>
<!-- JSON Processing API -->
<dependency>
<groupId>javax.json</groupId>
<artifactId>javax.json-api</artifactId>
<version>1.1.4</version>
</dependency>
<!-- Reference Implementation for JSON Processing -->
<dependency>
<groupId>org.glassfish</groupId>
<artifactId>javax.json</artifactId>
<version>1.1.4</version>
</dependency>
</dependencies>
```
import语句:
```java
import javax.json.Json;
import javax.json.JsonObject;
import javax.json.JsonReader;
import org.eclipse.californium.core.CoapClient;
import org.eclipse.californium.core.CoapResponse;
import org.eclipse.paho.client.mqttv3.MqttClient;
import org.eclipse.paho.client.mqttv3.MqttException;
import org.eclipse.paho.client.mqttv3.MqttMessage;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.integration.annotation.ServiceActivator;
import org.springframework.messaging.Message;
import org.springframework.messaging.MessagingException;
import org.springframework.messaging.handler.annotation.MessageMapping;
import org.springframework.messaging.simp.SimpMessagingTemplate;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
```
完整代码如下:
SpringBootMqttCoapIotPlatformApplication.java
```java
@Configuration
@RestController
public class SpringBootMqttCoapIotPlatformApplication {
@Autowired
private SimpMessagingTemplate messagingTemplate;
@PostMapping("/http")
public ResponseEntity<String> handleHttpPostRequest(@RequestBody String requestBody) {
JsonObject json = readJsonObject(requestBody);
String message = json.getString("message");
messagingTemplate.convertAndSend("/topic/http", message);
return new ResponseEntity<>("Message sent to HTTP topic", HttpStatus.OK);
}
@MessageMapping("/mqtt")
public void handleMqttMessage(String message) throws MqttException {
MqttClient client = new MqttClient("tcp://localhost:1883", MqttClient.generateClientId());
client.connect();
MqttMessage mqttMessage = new MqttMessage();
mqttMessage.setPayload(message.getBytes());
client.publish("mqtt", mqttMessage);
client.disconnect();
}
@ServiceActivator(inputChannel = "coapChannel")
public void handleCoapMessage(Message<?> message) {
CoapClient client = new CoapClient("coap://localhost:5683/iotsensors");
CoapResponse response = client.post(message.getPayload().toString(), 0);
String responseBody = response.getResponseText();
messagingTemplate.convertAndSend("/topic/coap", responseBody);
}
@GetMapping("/coap/{payload}")
public ResponseEntity<String> handleHttpGetRequest(@PathVariable String payload) {
CoapClient client = new CoapClient("coap://localhost:5683/iotsensors");
CoapResponse response = client.post(payload, 0);
return new ResponseEntity<>(response.getResponseText(), HttpStatus.OK);
}
@Bean
public CoapInboundGateway coapInboundGateway() {
CoapInboundGateway coapInboundGateway = new CoapInboundGateway("/iotsensors");
coapInboundGateway.setRequestChannel(coapChannel());
return coapInboundGateway;
}
@Bean
public MessageChannel coapChannel() {
return new DirectChannel();
}
private JsonObject readJsonObject(String json) {
JsonReader reader = Json.createReader(new StringReader(json));
return reader.readObject();
}
public static void main(String[] args) {
SpringApplication.run(SpringBootMqttCoapIotPlatformApplication.class, args);
}
}
```
CoapInboundGateway.java
```java
public class CoapInboundGateway extends AbstractInboundGateway {
private CoapServer coapServer;
private String uri;
public CoapInboundGateway(String uri) {
this.coapServer = new CoapServer();
this.uri = uri;
}
public void setRequestChannel(MessageChannel requestChannel) {
super.setRequestChannel(requestChannel);
}
public void start() {
coapServer.add(new CoapResource(uri) {
@Override
public void handlePOST(CoapExchange exchange) {
Message<?> message = createMessage(exchange.getRequestText());
if (message != null) {
send(message);
}
}
});
coapServer.start();
}
public void stop() {
coapServer.stop();
}
private Message<?> createMessage(String payload) {
if (!StringUtils.isEmpty(payload)) {
return getMessageBuilderFactory().withPayload(payload).build();
}
return null;
}
}
```
application.properties
```properties
spring.application.name=Spring Boot IoT Platform
spring.main.banner-mode=off
server.port=8080
```
CoapConfiguration.java
```java
@Configuration
public class CoapConfiguration {
@Autowired
private CoapInboundGateway coapInboundGateway;
@Bean
public CoapEndpoint coapEndpoint() {
return new CoapEndpoint(5683);
}
@Bean
public CoapServer coapServer() {
CoapServer coapServer = new CoapServer();
coapServer.addEndpoint(coapEndpoint());
coapServer.add(coapInboundGateway);
coapServer.start();
return coapServer;
}
@PreDestroy
public void stopCoapServer() {
coapServer().stop();
}
}
```
注意:示范代码只是演示如何使用Spring Boot设计物联网平台,示例代码中的MQTT和CoAP服务器均为本地服务器,并且没有进行安全验证。在实际应用中,需要根据实际需求做出相应的配置和修改。
相关推荐
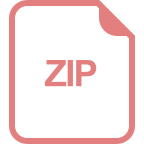
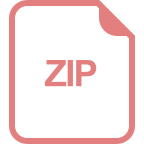
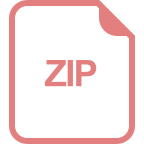














