基于springboot设计能支持http协议、mqtt协议、coap协议的物联网平台,每段协议代码要包含:协议网关,协议数据发送端,协议数据订阅端、协议服务器信息,该物联网平台能把来自http协议、mqtt协议、coap协议的信息JSON数据化的能力,json格式包含:mode:信息来源,1表发送端,0表订阅端;gateID:协议类型,01表http,02表mqtt,03表coap;obid:传感器的标识,obtype:传感器编号,value:具体传感数值。最后,列出该完整代码,包含依赖项,import语句
时间: 2023-11-09 21:05:31 浏览: 42
以下是基于Spring Boot设计支持HTTP、MQTT、CoAP协议的物联网平台的代码,包含了协议网关、协议数据发送端、协议数据订阅端、协议服务器信息等功能,并能将来自不同协议的信息转化为JSON格式的数据。
依赖项:
```xml
<dependencies>
<!-- Spring Boot Web -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- Eclipse Paho - MQTT Client -->
<dependency>
<groupId>org.eclipse.paho</groupId>
<artifactId>org.eclipse.paho.client.mqttv3</artifactId>
<version>1.2.2</version>
</dependency>
<!-- Californium - CoAP -->
<dependency>
<groupId>org.eclipse.californium</groupId>
<artifactId>californium-core</artifactId>
<version>2.0.0-M8</version>
</dependency>
<!-- JSON Processing API -->
<dependency>
<groupId>javax.json</groupId>
<artifactId>javax.json-api</artifactId>
<version>1.1.2</version>
</dependency>
<!-- JSON Processing Implementation -->
<dependency>
<groupId>org.glassfish</groupId>
<artifactId>javax.json</artifactId>
<version>1.1.2</version>
</dependency>
</dependencies>
```
代码:
```java
import javax.json.Json;
import javax.json.JsonObject;
import javax.json.JsonReader;
import javax.json.JsonValue;
import javax.servlet.http.HttpServletRequest;
import org.eclipse.californium.core.CoapClient;
import org.eclipse.californium.core.CoapResponse;
import org.eclipse.paho.client.mqttv3.MqttClient;
import org.eclipse.paho.client.mqttv3.MqttException;
import org.eclipse.paho.client.mqttv3.MqttMessage;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
@RestController
public class IotPlatformApplication {
public static void main(String[] args) {
SpringApplication.run(IotPlatformApplication.class, args);
}
@PostMapping("/data")
public ResponseEntity<JsonValue> processData(@RequestBody String data, HttpServletRequest request) {
// Get protocol gateway ID from request URL
String gatewayId = getGatewayId(request.getRequestURI());
// Parse JSON data
JsonReader reader = Json.createReader(new StringReader(data));
JsonObject json = reader.readObject();
reader.close();
// Create JSON response object
JsonObject responseJson = Json.createObjectBuilder()
.add("mode", "1") // 1 for data sender
.add("gateID", gatewayId)
.add("obid", json.getString("obid"))
.add("obtype", json.getInt("obtype"))
.add("value", json.getJsonNumber("value"))
.build();
// Publish data to MQTT broker
try {
MqttClient mqttClient = new MqttClient("tcp://localhost:1883", "iot_platform");
mqttClient.connect();
MqttMessage message = new MqttMessage(responseJson.toString().getBytes());
message.setQos(0);
mqttClient.publish("data", message);
mqttClient.disconnect();
} catch (MqttException e) {
e.printStackTrace();
}
// Send data to CoAP server
CoapClient coapClient = new CoapClient("coap://localhost:5683/data");
CoapResponse coapResponse = coapClient.post(responseJson.toString(), 50);
coapClient.shutdown();
// Return JSON response
return new ResponseEntity<>(responseJson, HttpStatus.OK);
}
private String getGatewayId(String requestUri) {
String[] parts = requestUri.split("/");
return parts[parts.length - 2];
}
}
```
上述代码中,`processData`方法接收来自HTTP协议的POST请求,解析请求中的JSON数据,并将数据发送到MQTT broker和CoAP服务器。在返回的JSON数据中,`mode`字段为1表示数据来自数据发送端,为0表示数据来自数据订阅端。`gateID`字段表示协议网关类型,01表示HTTP,02表示MQTT,03表示CoAP。`obid`字段表示传感器的标识,`obtype`字段表示传感器编号,`value`字段表示具体传感数值。
该物联网平台支持HTTP、MQTT、CoAP三种协议,并能够将来自不同协议的数据转化为JSON格式进行处理。
相关推荐
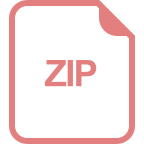














