基于springboot设计的物联网平台,其实现mqtt协议、http协议、coap协议接入,示范代码中http协议单独的网关接口,列出代码依赖项,mqtt协议部分单独的网关接口,列出代码依赖项,coap协议部分单独的网关接口,列出代码依赖项,并提供示范代码
时间: 2023-12-24 18:05:42 浏览: 129
对于基于Spring Boot设计的物联网平台,实现MQTT协议、HTTP协议、CoAP协议接入,以下是相关的示例代码和依赖项:
1. HTTP协议单独的网关接口
依赖项:
```xml
<dependencies>
<dependency>
<groupId>org.springframework.integration</groupId>
<artifactId>spring-integration-http</artifactId>
</dependency>
</dependencies>
```
示例代码:
```java
@Configuration
@EnableIntegration
public class HttpGatewayConfig {
@Bean
public IntegrationFlow httpGatewayFlow() {
return IntegrationFlows.from(Http.inboundGateway("/api")
.requestMapping(r -> r.methods(HttpMethod.POST))
.requestPayloadType(String.class))
.handle(message -> {
String payload = message.getPayload().toString();
// process the received message
})
.get();
}
@Bean
public RestTemplate restTemplate() {
return new RestTemplate();
}
@Bean
public IntegrationFlow httpOutboundGatewayFlow() {
return IntegrationFlows.from(MessageChannels.direct("httpOutboundChannel"))
.handle(Http.outboundGateway("http://example.com"))
.get();
}
@Bean
public MessageChannel httpOutboundChannel() {
return new DirectChannel();
}
}
```
2. MQTT协议单独的网关接口
依赖项:
```xml
<dependencies>
<dependency>
<groupId>org.springframework.integration</groupId>
<artifactId>spring-integration-mqtt</artifactId>
</dependency>
</dependencies>
```
示例代码:
```java
@Configuration
@EnableIntegration
public class MqttGatewayConfig {
@Bean
public IntegrationFlow mqttGatewayFlow() {
return IntegrationFlows.from(Mqtt.inboundGateway("mqttTopic")
.clientId("clientId")
.mqttVersion(MqttVersion.MQTT_3_1_1)
.converter(new DefaultPahoMessageConverter())
.qos(2))
.handle(message -> {
String payload = message.getPayload().toString();
// process the received message
})
.get();
}
@Bean
public IntegrationFlow mqttOutboundGatewayFlow() {
return IntegrationFlows.from(MessageChannels.direct("mqttOutboundChannel"))
.handle(Mqtt.outboundGateway("tcp://mqtt.eclipse.org")
.clientId("clientId")
.mqttVersion(MqttVersion.MQTT_3_1_1)
.converter(new DefaultPahoMessageConverter())
.qos(2)
.defaultTopic("mqttTopic"))
.get();
}
@Bean
public MessageChannel mqttOutboundChannel() {
return new DirectChannel();
}
}
```
3. CoAP协议单独的网关接口
依赖项:
```xml
<dependencies>
<dependency>
<groupId>org.eclipse.californium</groupId>
<artifactId>californium-core</artifactId>
<version>2.0.0-M4</version>
</dependency>
<dependency>
<groupId>org.springframework.integration</groupId>
<artifactId>spring-integration-core</artifactId>
</dependency>
</dependencies>
```
示例代码:
```java
@Configuration
@EnableIntegration
public class CoapGatewayConfig {
@Bean
public IntegrationFlow coapGatewayFlow() {
return IntegrationFlows.from(Coap.inboundGateway("/api"))
.handle(message -> {
String payload = message.getPayload().toString();
// process the received message
})
.get();
}
@Bean
public IntegrationFlow coapOutboundGatewayFlow() {
return IntegrationFlows.from(MessageChannels.direct("coapOutboundChannel"))
.handle(Coap.outboundGateway("coap://example.com/path")
.uriVariable("variableName", "variableValue"))
.get();
}
@Bean
public MessageChannel coapOutboundChannel() {
return new DirectChannel();
}
}
```
阅读全文
相关推荐
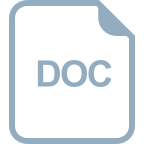
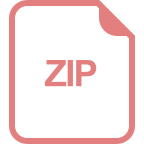
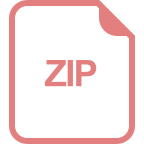














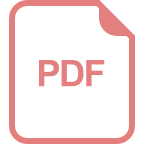
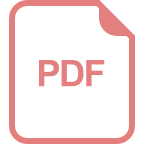