public class TestClock extends JFrame { private String AorP; private String hour; private String minute; private String second; public TestClock() { this.setSize(1000, 800); this.setVisible(true); this.setDefaultCloseOperation(EXIT_ON_CLOSE); this.setLayout(new GridLayout(2, 1)); Panel p1 = new Panel(new GridLayout(1, 3)); while (true) { Calendar c = Calendar.getInstance(); hour = Integer.toString(c.get(Calendar.HOUR)); minute = Integer.toString(c.get(Calendar.MINUTE)); second = Integer.toString(c.get(Calendar.SECOND)); AorP = Integer.toString(c.get(Calendar.AM_PM)); JLabel HH = new JLabel(hour, SwingConstants.CENTER); JLabel MM = new JLabel(minute, SwingConstants.CENTER); JLabel SS = new JLabel(second, SwingConstants.CENTER); JLabel AorP = new JLabel("AM/PM", SwingConstants.CENTER); HH.setFont(new Font(Font.SERIF, Font.ITALIC, 36)); MM.setFont(new Font(Font.SERIF, Font.ITALIC, 36)); SS.setFont(new Font(Font.SERIF, Font.ITALIC, 36)); AorP.setFont(new Font(Font.SERIF, Font.ITALIC, 26)); p1.add(HH); p1.add(MM); p1.add(SS); this.add(p1); this.add(AorP); } } public static void main(String[] args) { // TODO Auto-generated method stub TestClock clock = new TestClock(); } }为什么不显示
时间: 2024-02-04 07:01:55 浏览: 24
这段代码可能会导致程序卡死,因为while(true)会让程序无限循环下去,而且在while循环中不断地创建和添加标签,可能会导致内存溢出。这会导致程序无法正常运行,因此窗口不会显示。
如果想要实现一个实时显示当前时间的时钟,可以使用Timer类来实现。Timer类可以在指定时间间隔内重复执行指定任务,这样就可以实现每隔一段时间更新一次时钟的效果。具体实现可以参考以下代码:
```java
import java.awt.Font;
import java.awt.GridLayout;
import java.util.Calendar;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.SwingConstants;
import javax.swing.Timer;
public class TestClock extends JFrame {
private JLabel hourLabel;
private JLabel minuteLabel;
private JLabel secondLabel;
private JLabel amPmLabel;
public TestClock() {
this.setSize(300, 150);
this.setDefaultCloseOperation(EXIT_ON_CLOSE);
this.setLayout(new GridLayout(2, 1));
JPanel timePanel = new JPanel(new GridLayout(1, 3));
hourLabel = new JLabel("00", SwingConstants.CENTER);
minuteLabel = new JLabel("00", SwingConstants.CENTER);
secondLabel = new JLabel("00", SwingConstants.CENTER);
timePanel.add(hourLabel);
timePanel.add(minuteLabel);
timePanel.add(secondLabel);
this.add(timePanel);
amPmLabel = new JLabel("AM", SwingConstants.CENTER);
amPmLabel.setFont(new Font(Font.SERIF, Font.ITALIC, 24));
this.add(amPmLabel);
Timer timer = new Timer(1000, e -> {
Calendar calendar = Calendar.getInstance();
int hour = calendar.get(Calendar.HOUR_OF_DAY);
int minute = calendar.get(Calendar.MINUTE);
int second = calendar.get(Calendar.SECOND);
String amPm = hour < 12 ? "AM" : "PM";
hour = hour % 12;
hourLabel.setText(String.format("%02d", hour));
minuteLabel.setText(String.format("%02d", minute));
secondLabel.setText(String.format("%02d", second));
amPmLabel.setText(amPm);
});
timer.start();
}
public static void main(String[] args) {
TestClock clock = new TestClock();
clock.setVisible(true);
}
}
```
这个时钟每隔1秒更新一次,实时显示当前时间。注意,这里用到了Java 8中的Lambda表达式来简化代码。
相关推荐
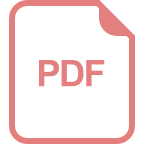
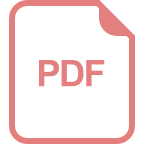
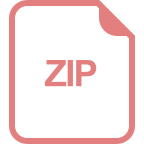














