public class TestClock extends JFrame { private String aorp; private String hour; private String minute; private String second; private Panel p1; public TestClock() { super("time"); this.setSize(1500, 1300); this.setVisible(true); this.setDefaultCloseOperation(EXIT_ON_CLOSE); this.setLayout(new GridLayout(2, 1)); p1 = new Panel(new GridLayout(1, 3)); JLabel HH = new JLabel(hour, SwingConstants.CENTER); JLabel MM = new JLabel(minute, SwingConstants.CENTER); JLabel SS = new JLabel(second, SwingConstants.CENTER); JLabel AorP = new JLabel(aorp, SwingConstants.CENTER); HH.setFont(new Font(Font.SERIF+":", Font.ITALIC, 36)); MM.setFont(new Font(Font.SERIF+":", Font.ITALIC, 36)); SS.setFont(new Font(Font.SERIF, Font.ITALIC, 36)); AorP.setFont(new Font(Font.SERIF, Font.ITALIC, 26)); p1.add(HH); p1.add(MM); p1.add(SS); this.add(p1); this.add(AorP); this.repaint(); HH.setText(hour); MM.setText(minute); SS.setText(second); AorP.setText(aorp); } public void showTime() { Calendar c = Calendar.getInstance(); hour = Integer.toString(c.get(Calendar.HOUR)); minute = Integer.toString(c.get(Calendar.MINUTE)); second = Integer.toString(c.get(Calendar.SECOND)); aorp = Integer.toString(c.get(Calendar.AM_PM)); } public static void main(String[] args) { // TODO Auto-generated method stub TestClock clock = new TestClock(); while (true) { clock.showTime(); try { Thread.sleep(1000); } catch (InterruptedException e) { e.printStackTrace(); } } } }
时间: 2024-02-01 08:02:19 浏览: 22
在你的代码中,`TestClock` 的构造方法中已经将 `JLabel` 对象添加到了窗口中,但是你在 `main` 方法中也添加了一个 `while(true)` 循环,这可能会导致窗口无法正常显示。因此,你应该将 `while(true)` 循环删除,并使用 `Timer` 对象来定时更新窗口中的时间显示。
具体来说,你可以在 `TestClock` 的构造方法中添加以下代码:
```
Timer t = new Timer(1000, new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
showTime();
HH.setText(hour);
MM.setText(minute);
SS.setText(second);
AorP.setText(aorp);
}
});
t.start();
```
这样,每隔一秒钟,`Timer` 对象就会调用 `showTime()` 方法来更新时间信息,并将更新后的时间信息显示在窗口中。同时,你也需要将 `while(true)` 循环删除,并将 `TestClock` 对象的创建放在 `main` 方法中。
完整代码如下:
```
public class TestClock extends JFrame {
private String aorp;
private String hour;
private String minute;
private String second;
private Panel p1;
public TestClock() {
super("time");
this.setSize(1500, 1300);
this.setVisible(true);
this.setDefaultCloseOperation(EXIT_ON_CLOSE);
this.setLayout(new GridLayout(2, 1));
p1 = new Panel(new GridLayout(1, 3));
JLabel HH = new JLabel(hour, SwingConstants.CENTER);
JLabel MM = new JLabel(minute, SwingConstants.CENTER);
JLabel SS = new JLabel(second, SwingConstants.CENTER);
JLabel AorP = new JLabel(aorp, SwingConstants.CENTER);
HH.setFont(new Font(Font.SERIF + ":", Font.ITALIC, 36));
MM.setFont(new Font(Font.SERIF + ":", Font.ITALIC, 36));
SS.setFont(new Font(Font.SERIF, Font.ITALIC, 36));
AorP.setFont(new Font(Font.SERIF, Font.ITALIC, 26));
p1.add(HH);
p1.add(MM);
p1.add(SS);
this.add(p1);
this.add(AorP);
this.repaint();
HH.setText(hour);
MM.setText(minute);
SS.setText(second);
AorP.setText(aorp);
Timer t = new Timer(1000, new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
showTime();
HH.setText(hour);
MM.setText(minute);
SS.setText(second);
AorP.setText(aorp);
}
});
t.start();
}
public void showTime() {
Calendar c = Calendar.getInstance();
hour = Integer.toString(c.get(Calendar.HOUR));
minute = Integer.toString(c.get(Calendar.MINUTE));
second = Integer.toString(c.get(Calendar.SECOND));
aorp = Integer.toString(c.get(Calendar.AM_PM));
}
public static void main(String[] args) {
TestClock clock = new TestClock();
}
}
```
尝试运行这个程序,你就可以看到窗口中正确显示当前的时间了。
相关推荐
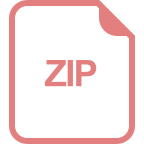
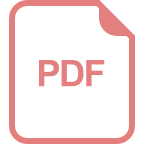
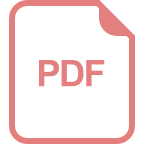















