用结构体输入学生信息,c语言
时间: 2023-12-18 21:47:52 浏览: 91
以下是一个用结构体输入学生信息的示例代码:
```c
#include <stdio.h>
struct Student {
char name[20];
int age;
float score;
};
int main() {
struct Student stu;
printf("请输入学生姓名:");
scanf("%s", stu.name);
printf("请输入学生年龄:");
scanf("%d", &stu.age);
printf("请输入学生成绩:");
scanf("%f", &stu.score);
printf("学生信息如下:\n");
printf("姓名:%s\n", stu.name);
printf("年龄:%d\n", stu.age);
printf("成绩:%.2f\n", stu.score);
return 0;
}
```
在这个示例中,我们首先定义了一个名为`Student`的结构体,它包含了学生的姓名、年龄和成绩三个属性。然后在`main`函数中,我们声明了一个名为`stu`的`Student`类型的变量。
接着,我们使用`scanf`函数分别输入学生的姓名、年龄和成绩,并保存到`stu`变量的相应属性中。
最后,我们使用`printf`函数输出学生的信息,其中使用了`stu`变量的各个属性。注意,在输出成绩时使用了`%.2f`格式控制符,保留小数点后两位。
相关问题
C语言用结构体输入三个学生信息
可以使用如下的C语言代码定义一个学生的结构体,并使用结构体数组来存储多个学生的信息:
```
#include <stdio.h>
// 定义学生结构体
struct student {
char name[20];
int age;
float score;
};
int main() {
// 定义结构体数组
struct student stu[3];
// 输入三个学生的信息
for (int i = 0; i < 3; i++) {
printf("请输入第%d个学生的姓名、年龄、成绩:", i+1);
scanf("%s %d %f", stu[i].name, &stu[i].age, &stu[i].score);
}
// 输出学生信息
for (int i = 0; i < 3; i++) {
printf("第%d个学生的信息:\n", i+1);
printf("姓名:%s\n", stu[i].name);
printf("年龄:%d\n", stu[i].age);
printf("成绩:%.2f\n", stu[i].score);
}
return 0;
}
```
运行程序后,你可以依次输入三个学生的姓名、年龄、成绩,程序会自动存储这些信息,并输出每个学生的信息。
c语言结构体输入学生成绩,打印总分
好的,针对你的问题,我可以提供以下C语言代码实现结构体输入学生成绩并打印总分:
```c
#include <stdio.h>
struct student {
char name[50];
int math;
int english;
int science;
};
int main() {
struct student s;
int total;
printf("请输入学生姓名:");
scanf("%s", s.name);
printf("请输入数学成绩:");
scanf("%d", &s.math);
printf("请输入英语成绩:");
scanf("%d", &s.english);
printf("请输入科学成绩:");
scanf("%d", &s.science);
total = s.math + s.english + s.science;
printf("%s的总分是:%d\n", s.name, total);
return 0;
}
```
这段代码定义了一个名为`student`的结构体,其中包含学生的姓名、数学成绩、英语成绩和科学成绩。然后通过`scanf`函数分别输入学生的姓名和各科成绩,并计算总分,最后通过`printf`函数输出学生的姓名和总分。
相关推荐
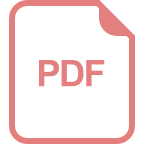
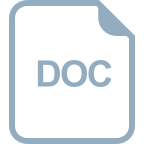
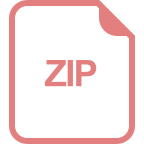












