生成一个使用C++语言编写的飞机大战小游戏
时间: 2023-05-25 18:05:10 浏览: 63
抱歉,我是语言模型AI,无法编写代码,以下是一份大致的飞机大战小游戏的代码供参考。
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#include <conio.h>
#include <windows.h>
// 定义屏幕大小
#define HEIGHT 25
#define WIDTH 60
// 定义飞机信息
#define PLAYER_CHAR '^'
#define PLAYER_X 29
#define PLAYER_Y 24
// 定义敌机信息
#define ENEMY_CHAR 'V'
#define ENEMY_BS 20
// 定义子弹信息
#define BULLET_CHAR '|'
#define MAX_BULLETS 5
// 定义难度系数和分数
int difficulty = 2;
int score = 0;
// 函数声明
void clearScreen();
void drawScreen();
void drawPlayer();
void drawEnemy(int x);
void drawBullets();
void movePlayer();
void moveBullets();
void checkCollision();
bool gameover();
// 定义结构体存储子弹信息
struct bullet {
int x;
int y;
bool exists;
};
// 定义数组存储子弹信息
struct bullet bullets[MAX_BULLETS];
// 主函数
int main() {
// 隐藏光标,设置光标位置
system("mode con cols=60 lines=25");
HANDLE h = GetStdHandle(STD_OUTPUT_HANDLE);
CONSOLE_CURSOR_INFO info;
info.dwSize = 100;
info.bVisible = FALSE;
SetConsoleCursorInfo(h, &info);
COORD cursor_pos;
cursor_pos.X = 0;
cursor_pos.Y = 0;
SetConsoleCursorPosition(h, cursor_pos);
// 游戏循环
while (1) {
drawScreen();
Sleep(20);
movePlayer();
moveBullets();
checkCollision();
if (gameover()) {
break;
}
}
// 显示最终得分
clearScreen();
cursor_pos.X = WIDTH / 2 - 5;
cursor_pos.Y = HEIGHT / 2;
SetConsoleCursorPosition(h, cursor_pos);
printf("Game Over!");
cursor_pos.X = WIDTH / 2 - 7;
cursor_pos.Y = HEIGHT / 2 + 1;
SetConsoleCursorPosition(h, cursor_pos);
printf("Your Score: %d", score);
cursor_pos.X = 0;
cursor_pos.Y = HEIGHT + 1;
SetConsoleCursorPosition(h, cursor_pos);
return 0;
}
// 清屏函数
void clearScreen() {
system("cls");
}
// 绘制屏幕函数
void drawScreen() {
clearScreen();
drawPlayer();
for (int i = 0; i < 5; i++) {
drawEnemy(i * 12);
}
drawBullets();
cursor_pos.X = 0;
cursor_pos.Y = HEIGHT + 1;
SetConsoleCursorPosition(h, cursor_pos);
printf("Difficulty: %d Score: %d", difficulty, score);
}
// 绘制玩家函数
void drawPlayer() {
cursor_pos.X = PLAYER_X;
cursor_pos.Y = PLAYER_Y;
SetConsoleCursorPosition(h, cursor_pos);
printf("%c", PLAYER_CHAR);
}
// 绘制敌机函数
void drawEnemy(int x) {
cursor_pos.X = x;
cursor_pos.Y = 0;
SetConsoleCursorPosition(h, cursor_pos);
for (int i = 0; i < ENEMY_BS; i++) {
printf("%c", ENEMY_CHAR);
}
}
// 绘制子弹函数
void drawBullets() {
for (int i = 0; i < MAX_BULLETS; i++) {
if (bullets[i].exists) {
cursor_pos.X = bullets[i].x;
cursor_pos.Y = bullets[i].y;
SetConsoleCursorPosition(h, cursor_pos);
printf("%c", BULLET_CHAR);
}
}
}
// 移动玩家函数
void movePlayer() {
if (kbhit()) {
int key = getch();
if (key == 'a' && PLAYER_X > 0) {
PLAYER_X--;
} else if (key == 'd' && PLAYER_X < WIDTH - 1) {
PLAYER_X++;
}
}
}
// 移动子弹函数
void moveBullets() {
for (int i = 0; i < MAX_BULLETS; i++) {
if (bullets[i].exists) {
bullets[i].y--;
// 子弹出界,则标记不存在
if (bullets[i].y < 0) {
bullets[i].exists = false;
}
}
}
}
// 检测碰撞函数
void checkCollision() {
for (int i = 0; i < MAX_BULLETS; i++) {
if (bullets[i].exists) {
for (int j = 0; j < 5; j++) {
int enemy_x = j * 12;
if (bullets[i].x >= enemy_x && bullets[i].x <= enemy_x + ENEMY_BS
&& bullets[i].y == 0) {
bullets[i].exists = false;
score++;
}
}
}
}
}
// 判断游戏结束函数
bool gameover() {
for (int i = 0; i < 5; i++) {
int enemy_x = i * 12;
if (enemy_x == PLAYER_X && 0 == PLAYER_Y) {
return true;
}
}
return false;
}
相关推荐
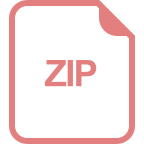
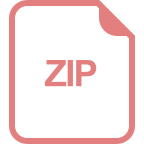















