Write a program to read the file Harry_Potter.txt and count the number of words in this file. Then, create and write the number of words in another .txt file named 'Count_Words' (You do not have to submit the file you generate.) Download the Harry Potter.txt file.
时间: 2023-11-27 18:50:25 浏览: 37
Here is a Python program that reads the file 'Harry_Potter.txt', counts the number of words in it, and writes the count to a new file 'Count_Words.txt':
```python
# open the Harry Potter text file
with open('Harry_Potter.txt', 'r') as file:
# read the contents of the file
text = file.read()
# split the text into words
words = text.split()
# count the number of words
num_words = len(words)
# create a new file and write the number of words
with open('Count_Words.txt', 'w') as file:
file.write(str(num_words))
```
This program first opens the 'Harry_Potter.txt' file and reads its contents into a variable called `text`. It then splits the text into individual words using the `split()` method and counts the number of words using the `len()` function.
Finally, the program creates a new file called 'Count_Words.txt' using the `open()` function with the `w` (write) mode. It writes the number of words to the file using the `write()` method, converting the integer to a string using the `str()` function.
Note that you need to make sure the 'Harry_Potter.txt' file is in the same directory as the Python program for this to work.
相关推荐
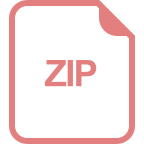
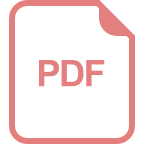















