哲学家进餐问题奇偶方法代码
时间: 2023-07-15 17:13:55 浏览: 118
下面是哲学家进餐问题的奇偶方法的示例代码(使用Java语言实现):
```
import java.util.concurrent.Semaphore;
public class DiningPhilosophers {
private static final int NUM_PHILOSOPHERS = 5;
private static final Semaphore[] forks = new Semaphore[NUM_PHILOSOPHERS];
private static final Semaphore oddPhilosophers = new Semaphore(1);
private static final Semaphore evenPhilosophers = new Semaphore(0);
public static void main(String[] args) {
for (int i = 0; i < NUM_PHILOSOPHERS; i++) {
forks[i] = new Semaphore(1);
}
for (int i = 0; i < NUM_PHILOSOPHERS; i++) {
int philosopherId = i;
new Thread(() -> {
while (true) {
think(philosopherId);
if (philosopherId % 2 == 0) {
evenPhilosophers.acquireUninterruptibly();
forks[philosopherId].acquireUninterruptibly();
forks[(philosopherId + 1) % NUM_PHILOSOPHERS].acquireUninterruptibly();
} else {
oddPhilosophers.acquireUninterruptibly();
forks[(philosopherId + 1) % NUM_PHILOSOPHERS].acquireUninterruptibly();
forks[philosopherId].acquireUninterruptibly();
}
eat(philosopherId);
if (philosopherId % 2 == 0) {
forks[(philosopherId + 1) % NUM_PHILOSOPHERS].release();
forks[philosopherId].release();
evenPhilosophers.release();
} else {
forks[philosopherId].release();
forks[(philosopherId + 1) % NUM_PHILOSOPHERS].release();
oddPhilosophers.release();
}
}
}).start();
}
}
private static void think(int philosopherId) {
System.out.println("Philosopher " + philosopherId + " is thinking.");
try {
Thread.sleep((long)(Math.random() * 10000));
} catch (InterruptedException e) {
e.printStackTrace();
}
}
private static void eat(int philosopherId) {
System.out.println("Philosopher " + philosopherId + " is eating.");
try {
Thread.sleep((long)(Math.random() * 10000));
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
```
这个实现中,oddPhilosophers和evenPhilosophers两个Semaphore用于控制奇数号和偶数号哲学家的交替就餐。在每个哲学家的线程中,首先进行思考(调用think()方法),然后根据自己的编号判断是奇数号还是偶数号哲学家,如果是偶数号哲学家,则先尝试获取右边的叉子(forks[philosopherId])和左边的叉子(forks[(philosopherId + 1) % NUM_PHILOSOPHERS]),如果获取成功,则开始就餐(调用eat()方法),然后释放叉子,唤醒下一个哲学家;否则,等待其他哲学家释放叉子。
如果是奇数号哲学家,则先尝试获取左边的叉子和右边的叉子,其余步骤与偶数号哲学家类似。
需要注意的是:奇偶方法只是一种解决哲学家进餐问题的方式,而不是唯一的正确方法。此外,哲学家进餐问题是一个经典的并发编程问题,涉及到很多细节和技巧,在实际应用中需要进行更加深入的研究和调试。
阅读全文
相关推荐
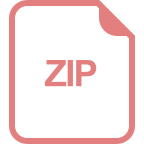
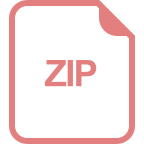

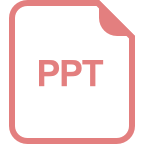
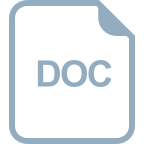
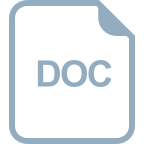
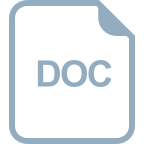
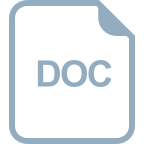

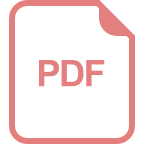
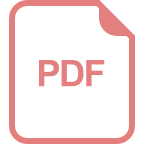
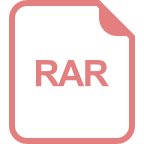



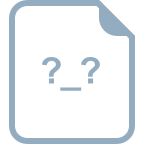