解决哲学家进餐问题可采用如下方法:a.仅当哲学家的左、右两只筷子均可用时,才允许他拿起筷子进餐;b.至多只允许有4位哲学家同时去拿左边的筷子,最终能保证至少有一位哲学家能够进餐;c.规定奇数号哲学家先拿起他左手的筷子,然后再拿起他右手的筷子,而偶数号哲学家则先拿起他右手的筷子,然后再拿起他左手的筷子。方法a在示例程序中给出,请用方法b和c写出不会发生死锁的哲学家进餐程序。
时间: 2023-11-27 15:49:16 浏览: 37
方法b:
```python
import threading
class Philosopher(threading.Thread):
def __init__(self, left_chopstick, right_chopstick, semaphore):
threading.Thread.__init__(self)
self.left_chopstick = left_chopstick
self.right_chopstick = right_chopstick
self.semaphore = semaphore
def run(self):
while True:
# 拿起左边的筷子,最多只有4个哲学家同时拿左边的筷子
self.semaphore.acquire()
self.left_chopstick.acquire()
# 拿起右边的筷子
self.right_chopstick.acquire()
# 进餐
print(f"{threading.current_thread().name} is eating...")
# 放下右边的筷子
self.right_chopstick.release()
# 放下左边的筷子,同时释放semaphore,允许其他哲学家拿起左边的筷子
self.left_chopstick.release()
self.semaphore.release()
if __name__ == "__main__":
chopsticks = [threading.Lock() for _ in range(5)]
semaphore = threading.Semaphore(4)
philosophers = []
for i in range(5):
philosopher = Philosopher(chopsticks[i], chopsticks[(i+1)%5], semaphore)
philosopher.name = f"Philosopher {i}"
philosophers.append(philosopher)
for philosopher in philosophers:
philosopher.start()
```
方法c:
```python
import threading
class Philosopher(threading.Thread):
def __init__(self, left_chopstick, right_chopstick):
threading.Thread.__init__(self)
self.left_chopstick = left_chopstick
self.right_chopstick = right_chopstick
def run(self):
while True:
# 奇数号哲学家先拿左边的筷子
if threading.current_thread().name[-1] == '1' or threading.current_thread().name[-1] == '3':
self.left_chopstick.acquire()
self.right_chopstick.acquire()
# 偶数号哲学家先拿右边的筷子
else:
self.right_chopstick.acquire()
self.left_chopstick.acquire()
# 进餐
print(f"{threading.current_thread().name} is eating...")
# 放下右边的筷子
self.right_chopstick.release()
# 放下左边的筷子
self.left_chopstick.release()
if __name__ == "__main__":
chopsticks = [threading.Lock() for _ in range(5)]
philosophers = []
for i in range(5):
philosopher = Philosopher(chopsticks[i], chopsticks[(i+1)%5])
philosopher.name = f"Philosopher {i}"
philosophers.append(philosopher)
for philosopher in philosophers:
philosopher.start()
```
以上两种方法都能保证不会发生死锁。方法b通过限制同时拿左边筷子的哲学家数量来避免死锁,方法c则通过奇偶号哲学家的不同拿筷子顺序来避免死锁。
相关推荐
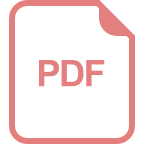
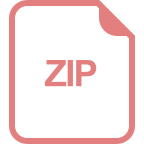














