二叉排序树。任意给定一组数据,设计一个算法,建立一棵二叉排序树,对它进行查找、插入、删除操作,用c语言实现
时间: 2024-03-05 14:51:03 浏览: 23
好的,我可以帮你解答这个问题。首先,我们来了解一下二叉排序树。
二叉排序树是一种特殊的二叉树,它的每个节点都满足以下条件:
1. 左子树中的所有节点的值都小于当前节点的值;
2. 右子树中的所有节点的值都大于当前节点的值;
3. 左子树和右子树也都是二叉排序树。
基于这个特性,我们可以在二叉排序树中实现快速的查找、插入和删除操作。
下面是一个基于 C 语言实现的二叉排序树:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义二叉排序树的节点结构体
typedef struct TreeNode {
int val;
struct TreeNode* left;
struct TreeNode* right;
} TreeNode;
// 查找节点
TreeNode* search(TreeNode* root, int val) {
if (root == NULL || root->val == val) {
return root;
}
if (val < root->val) {
return search(root->left, val);
} else {
return search(root->right, val);
}
}
// 插入节点
TreeNode* insert(TreeNode* root, int val) {
if (root == NULL) {
TreeNode* node = (TreeNode*)malloc(sizeof(TreeNode));
node->val = val;
node->left = NULL;
node->right = NULL;
return node;
}
if (val < root->val) {
root->left = insert(root->left, val);
} else if (val > root->val) {
root->right = insert(root->right, val);
}
return root;
}
// 删除节点
TreeNode* delete(TreeNode* root, int val) {
if (root == NULL) {
return NULL;
}
if (val < root->val) {
root->left = delete(root->left, val);
} else if (val > root->val) {
root->right = delete(root->right, val);
} else {
if (root->left == NULL) {
TreeNode* temp = root->right;
free(root);
return temp;
} else if (root->right == NULL) {
TreeNode* temp = root->left;
free(root);
return temp;
}
TreeNode* temp = root->right;
while (temp->left != NULL) {
temp = temp->left;
}
root->val = temp->val;
root->right = delete(root->right, temp->val);
}
return root;
}
// 打印二叉排序树
void printTree(TreeNode* root) {
if (root == NULL) {
return;
}
printf("%d ", root->val);
printTree(root->left);
printTree(root->right);
}
int main() {
// 初始化二叉排序树
TreeNode* root = NULL;
// 插入节点
root = insert(root, 5);
root = insert(root, 3);
root = insert(root, 7);
root = insert(root, 1);
root = insert(root, 9);
root = insert(root, 2);
// 打印二叉排序树
printf("Binary Search Tree: ");
printTree(root);
// 查找节点
TreeNode* node = search(root, 7);
if (node == NULL) {
printf("\n7 not found in the tree.\n");
} else {
printf("\n7 found in the tree.\n");
}
// 删除节点
root = delete(root, 5);
// 打印二叉排序树
printf("Binary Search Tree: ");
printTree(root);
return 0;
}
```
这个实现中,我们定义了一个 `TreeNode` 结构体,表示二叉排序树的节点。其中包含一个 `val` 属性表示节点的值,还有 `left` 和 `right` 两个指针,分别指向左子树和右子树。
接着,我们定义了 `search`、`insert` 和 `delete` 三个函数,分别实现了查找、插入和删除操作。其中,查找和插入操作比较简单,主要是递归实现。删除操作稍微复杂一些,因为需要考虑到删除节点后可能会破坏二叉排序树的结构,所以需要特殊处理。
最后,在 `main` 函数中,我们初始化了一棵二叉排序树,并对其进行了插入、查找和删除操作。
相关推荐
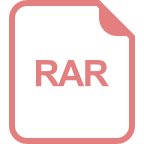
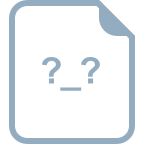
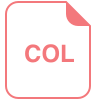
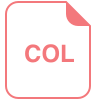
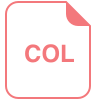
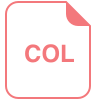
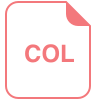









