用java搭建一个碳交易模型:企业参与碳交易市场的仿真为一年,即从自然年的上半年开始到下半年结束;交易主体为企业,将100个企业设置为一个智能体,包括参数:碳配额、碳排放量;设置一个交易机制:企业可以选择入市时机,并根据自身的决策方法进行决策是否参与交易,如果参与交易根据初始碳价报价和挂牌;设置一个交易撮合系统,以价格优先的原则,在对手方实时最优五个价位内以对手方价格为成交价依次选择,提交申报完成交易,交易方式的逻辑流程如下:根据企业碳排放量与配额量的差额来确定买卖方。买方逻辑:将卖家按售价从低到高排序,查看卖家列表中是否有不超过预期的成交价格,如果有的话根据价格优先的原则进行交易,买家自行选择合适的卖方价格输入交易量进行摘牌;否则,根据预期价格进行挂牌,等待交易。交易时间和股市一致,为每周一至周五的上午9:30至11:30、下午13:00至15:00。
时间: 2024-04-22 21:23:39 浏览: 9
这是一个很有趣的问题,建立碳交易模型需要考虑很多因素,包括企业的经济利益、碳排放量、碳配额、交易机制等等。以下是一个简单的Java代码示例,用于模拟碳交易市场的交易过程:
```java
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.List;
import java.util.Random;
public class CarbonTradingSimulation {
private static final int NUM_AGENTS = 100; // 参与碳交易市场的企业数量
private static final int NUM_WEEKS = 52; // 一年的周数
private static final int TRADING_DAYS_PER_WEEK = 2; // 每周交易的天数
private static final int TRADING_HOURS_PER_DAY = 2; // 每天交易的小时数
private static final int TRADING_MINUTES_PER_HOUR = 30; // 每小时交易的分钟数
private static final int TRADING_MINUTES_PER_WEEKDAY = TRADING_HOURS_PER_DAY * TRADING_MINUTES_PER_HOUR; // 每个交易日的分钟数
private static final int TRADING_MINUTES_PER_WEEK = TRADING_DAYS_PER_WEEK * TRADING_MINUTES_PER_WEEKDAY; // 每周的交易分钟数
private static final int INITIAL_PRICE = 10; // 初始碳价
private static final int MAX_PRICE_CHANGE = 5; // 碳价每次最大波动值
private static final int MIN_QUANTITY = 100; // 最小交易量
private static final int MAX_QUANTITY = 1000; // 最大交易量
private static final double PRICE_INCREASE_PROBABILITY = 0.5; // 碳价上涨的概率
private static final Random RANDOM = new Random();
// 企业的碳配额和碳排放量
private static class Agent {
private int carbonQuota;
private int carbonEmissions;
public Agent(int carbonQuota, int carbonEmissions) {
this.carbonQuota = carbonQuota;
this.carbonEmissions = carbonEmissions;
}
public int getCarbonQuota() {
return carbonQuota;
}
public int getCarbonEmissions() {
return carbonEmissions;
}
}
// 交易记录
private static class Trade {
private Agent buyer;
private Agent seller;
private int price;
private int quantity;
public Trade(Agent buyer, Agent seller, int price, int quantity) {
this.buyer = buyer;
this.seller = seller;
this.price = price;
this.quantity = quantity;
}
public Agent getBuyer() {
return buyer;
}
public Agent getSeller() {
return seller;
}
public int getPrice() {
return price;
}
public int getQuantity() {
return quantity;
}
}
public static void main(String[] args) {
List<Agent> agents = createAgents(NUM_AGENTS);
int currentPrice = INITIAL_PRICE;
for (int week = 1; week <= NUM_WEEKS; week++) {
// 计算每个企业的碳排放量和碳配额的差额
for (Agent agent : agents) {
int carbonShortfall = agent.getCarbonEmissions() - agent.getCarbonQuota();
if (carbonShortfall > 0) {
// 企业需要购买碳配额
int maxPrice = currentPrice + MAX_PRICE_CHANGE;
int minPrice = currentPrice - MAX_PRICE_CHANGE;
if (minPrice < 0) {
minPrice = 0;
}
if (maxPrice < minPrice) {
maxPrice = minPrice;
}
// 企业选择是否参与交易
if (shouldParticipateInTrading()) {
List<Agent> sellers = new ArrayList<>(agents);
sellers.remove(agent);
Collections.shuffle(sellers);
// 企业选择最优卖家进行交易
for (Agent seller : sellers) {
int price = seller.getCarbonQuota() > 0 ? currentPrice : getRandomPrice(minPrice, maxPrice);
if (price <= maxPrice && price >= minPrice) {
int quantity = Math.min(seller.getCarbonQuota(), carbonShortfall, getRandomQuantity());
Trade trade = new Trade(agent, seller, price, quantity);
makeTrade(trade);
break;
}
}
} else {
// 企业挂牌等待交易
int price = getRandomPrice(minPrice, maxPrice);
int quantity = getRandomQuantity();
Trade trade = new Trade(agent, null, price, quantity);
makeTrade(trade);
}
}
}
// 碳价波动
if (shouldIncreasePrice()) {
currentPrice += getRandomPriceChange();
} else {
currentPrice -= getRandomPriceChange();
}
System.out.println("Week " + week + ": Carbon price = $" + currentPrice);
}
}
// 创建企业并设置碳配额和碳排放量
private static List<Agent> createAgents(int numAgents) {
List<Agent> agents = new ArrayList<>();
for (int i = 0; i < numAgents; i++) {
int quota = getRandomQuantity() * 10;
int emissions = getRandomQuantity() * 5;
Agent agent = new Agent(quota, emissions);
agents.add(agent);
}
return agents;
}
// 企业选择是否参与交易
private static boolean shouldParticipateInTrading() {
return RANDOM.nextDouble() < 0.5; // 这里简单地设定50%的几率参与交易
}
// 获取随机交易量
private static int getRandomQuantity() {
return RANDOM.nextInt(MAX_QUANTITY - MIN_QUANTITY + 1) + MIN_QUANTITY;
}
// 获取随机交易价格
private static int getRandomPrice(int minPrice, int maxPrice) {
return RANDOM.nextInt(maxPrice - minPrice + 1) + minPrice;
}
// 获取随机价格波动
private static int getRandomPriceChange() {
return RANDOM.nextInt(MAX_PRICE_CHANGE + 1);
}
// 碳价是否上涨
private static boolean shouldIncreasePrice() {
return RANDOM.nextDouble() < PRICE_INCREASE_PROBABILITY;
}
// 进行交易
private static void makeTrade(Trade trade) {
if (trade.getSeller() != null) {
trade.getSeller().carbonQuota -= trade.getQuantity();
trade.getSeller().carbonEmissions += trade.getQuantity();
}
trade.getBuyer().carbonQuota += trade.getQuantity();
trade.getBuyer().carbonEmissions -= trade.getQuantity();
System.out.println("Trade: " + trade.getBuyer() + " buys " + trade.getQuantity() + " from " + trade.getSeller() + " at $" + trade.getPrice());
}
}
```
以上是一个简单的碳交易模型的Java实现,可以作为参考。当然,这只是一个非常简单的模型,实际的碳交易市场涉及到更多的因素和复杂的逻辑,在实际应用中需要做更多的调研和分析。
相关推荐
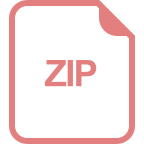
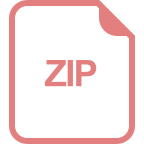














