stringRedisTemplate获取redis
时间: 2024-05-05 15:15:39 浏览: 72
StringRedisTemplate是Spring提供的一个用于操作Redis的工具类,主要用于操作字符串类型的数据。使用StringRedisTemplate可以方便地对Redis进行读写操作。
使用StringRedisTemplate获取redis数据的步骤如下:
1. 在Spring配置文件中配置StringRedisTemplate
```
<bean id="stringRedisTemplate" class="org.springframework.data.redis.core.StringRedisTemplate">
<property name="connectionFactory" ref="jedisConnectionFactory"/>
</bean>
```
2. 在Java代码中使用StringRedisTemplate获取redis数据
```
String value = stringRedisTemplate.opsForValue().get("key");
```
其中,`opsForValue()`方法返回一个`ValueOperations`对象,通过该对象可以对字符串类型的数据进行操作。`get()`方法用于获取指定key的value值。
可以根据需要,使用其他方法对redis进行操作,如set、incr等方法。
相关问题
stringRedisTemplate获取redis中hash的全部值,每次批量获取1000个,怎么写
你可以使用Redis的`scan`命令来实现分批获取hash中的全部值,具体步骤如下:
1. 使用`scan`命令获取hash中的全部field和value,每次获取1000个。可以使用`HashOperations`的`scan`方法来实现。
```
ScanOptions options = ScanOptions.scanOptions().count(1000).build();
Cursor<Map.Entry<Object, Object>> cursor = hashOperations.scan("hashKey", options);
```
2. 遍历游标,将field和value保存到一个Map中。
```
Map<Object, Object> resultMap = new HashMap<>();
while (cursor.hasNext()) {
Map.Entry<Object, Object> entry = cursor.next();
resultMap.put(entry.getKey(), entry.getValue());
}
```
3. 判断游标是否已经结束,如果未结束,则继续获取下一个批次的数据。如果已经结束,则返回保存了全部值的Map。
```
if (cursor.isClosed()) {
return resultMap;
} else {
options = options.cursor(cursor);
cursor = hashOperations.scan("hashKey", options);
}
```
完整代码示例如下:
```
public Map<Object, Object> getAllHashValues(String hashKey) {
HashOperations<String, Object, Object> hashOperations = stringRedisTemplate.opsForHash();
ScanOptions options = ScanOptions.scanOptions().count(1000).build();
Cursor<Map.Entry<Object, Object>> cursor = hashOperations.scan(hashKey, options);
Map<Object, Object> resultMap = new HashMap<>();
while (cursor.hasNext()) {
Map.Entry<Object, Object> entry = cursor.next();
resultMap.put(entry.getKey(), entry.getValue());
}
if (cursor.isClosed()) {
return resultMap;
} else {
options = options.cursor(cursor);
cursor = hashOperations.scan(hashKey, options);
while (cursor.hasNext()) {
Map.Entry<Object, Object> entry = cursor.next();
resultMap.put(entry.getKey(), entry.getValue());
}
return resultMap;
}
}
```
请注意,以上代码仅供参考,可能需要根据你的具体需求进行修改。
如何使用stringRedisTemplate去Redis中取一个List类型的数据
可以使用Spring Data Redis提供的`opsForList()`方法来获取`ListOperations`对象,然后调用该对象的`range()`方法来获取List数据。
示例代码如下:
```java
ListOperations<String, String> listOps = stringRedisTemplate.opsForList();
List<String> myList = listOps.range("myListKey", 0, -1);
```
其中,`myListKey`为Redis中存储List数据的Key。`range()`方法的第二个参数为起始下标,第三个参数为终止下标。如果想获取List中的所有元素,可以将第二个参数设置为0,将第三个参数设置为-1。
阅读全文
相关推荐
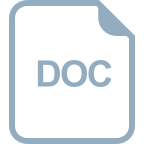
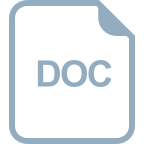
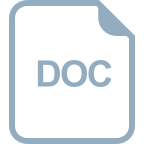


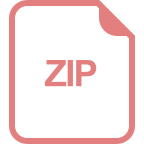
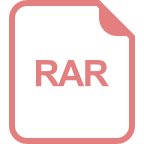









