写一下Sobel边缘检测算子对图像进行边缘检测运算的c程序代码。要求目标图像为灰度图像。
时间: 2024-04-04 08:32:54 浏览: 50
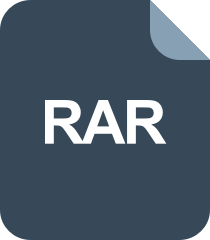
sobel-bianyuanjiance.rar_edge sobel_sobel_sobel算子_边缘检测
以下是使用Sobel边缘检测算子对灰度图像进行边缘检测的C代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <math.h>
#include "bmpfile.h"
#define PI 3.14159265358979323846
void sobel_edge_detection(unsigned char *src, unsigned char *dst, int width, int height)
{
int i, j, k, gx, gy, sum;
int sobel_x[3][3] = {{-1, 0, 1}, {-2, 0, 2}, {-1, 0, 1}};
int sobel_y[3][3] = {{-1, -2, -1}, {0, 0, 0}, {1, 2, 1}};
// 对图像进行边缘检测
for (i = 1; i < height-1; i++) {
for (j = 1; j < width-1; j++) {
gx = gy = 0;
for (k = 0; k < 3; k++) {
gx += src[(i-1)*width+(j-1+k)] * sobel_x[0][k];
gx += src[(i+1)*width+(j-1+k)] * sobel_x[2][k];
gy += src[(i-1)*width+(j-1+k)] * sobel_y[0][k];
gy += src[(i+1)*width+(j-1+k)] * sobel_y[2][k];
}
gx += src[i*width+(j-1)] * sobel_x[1][0];
gx += src[i*width+(j+1)] * sobel_x[1][2];
gy += src[i*width+(j-1)] * sobel_y[1][0];
gy += src[i*width+(j+1)] * sobel_y[1][2];
sum = abs(gx) + abs(gy);
sum = sum > 255 ? 255 : sum;
dst[i*width+j] = 255 - sum;
}
}
}
int main()
{
BMPFILEHEADER header;
BMPINFOHEADER info;
unsigned char *src, *dst;
int width, height, size;
FILE *fp;
// 读入BMP图像
fp = fopen("image.bmp", "rb");
fread(&header, sizeof(header), 1, fp);
fread(&info, sizeof(info), 1, fp);
width = info.biWidth;
height = info.biHeight;
size = width * height;
src = (unsigned char*)malloc(size);
dst = (unsigned char*)malloc(size);
fread(src, sizeof(unsigned char), size, fp);
fclose(fp);
// 对图像进行边缘检测
sobel_edge_detection(src, dst, width, height);
// 将结果保存为BMP图像
fp = fopen("result.bmp", "wb");
fwrite(&header, sizeof(header), 1, fp);
fwrite(&info, sizeof(info), 1, fp);
fwrite(dst, sizeof(unsigned char), size, fp);
fclose(fp);
// 释放内存
free(src);
free(dst);
return 0;
}
```
其中,`sobel_edge_detection()`函数用于对图像进行边缘检测,使用了Sobel算子计算水平方向和竖直方向上的梯度,并将边缘强度计算为两个方向上的梯度的绝对值之和,最终得到边缘检测结果。
阅读全文
相关推荐
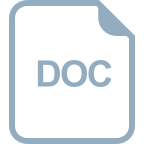
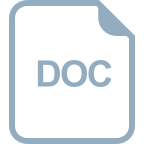

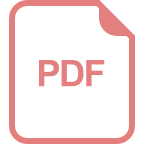
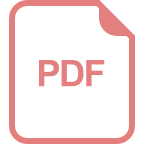
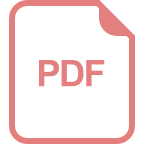
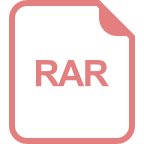
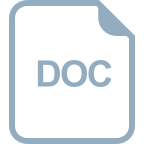
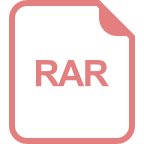
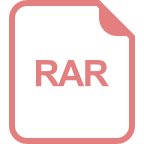
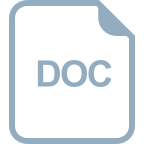
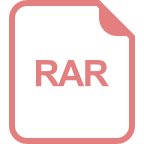
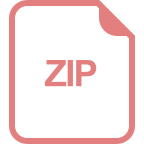
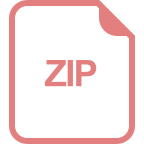
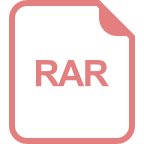
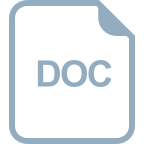
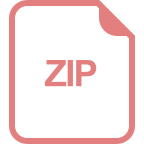
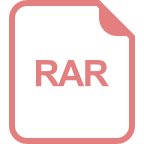