对文本文件内的每个单词都统计出其出现的次数; 按照每个单词出现次数的数量,降序排序。
时间: 2023-12-20 18:06:45 浏览: 68
可以使用Python来实现这个功能。具体步骤如下:
1. 读取文本文件,将文件内容保存到一个字符串变量中。
2. 将字符串变量中的标点符号和换行符等特殊字符去除,只保留单词。
3. 将字符串变量按照空格进行分割,得到单词列表。
4. 遍历单词列表,使用字典来统计每个单词出现的次数。
5. 将字典按照单词出现次数的数量进行降序排序。
6. 输出排序后的结果。
以下是Python代码实现:
```python
import re
# 读取文本文件
with open('file.txt', 'r') as f:
content = f.read()
# 去除特殊字符,只保留单词,转换为小写
content = re.sub(r'[^\w\s]', '', content).lower()
# 分割单词
words = content.split()
# 统计单词出现次数
word_count = {}
for word in words:
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
# 按照单词出现次数排序
sorted_word_count = sorted(word_count.items(), key=lambda x: x[1], reverse=True)
# 输出结果
for word, count in sorted_word_count:
print(f'{word}: {count}')
```
其中,`re.sub(r'[^\w\s]', '', content)` 用于去除特殊字符,`split()` 用于按照空格进行分割,`sorted()` 用于排序。
相关推荐
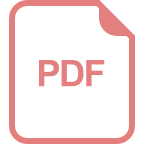
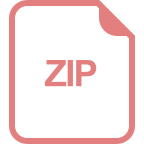
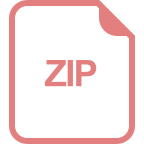















