html 转word python
时间: 2023-11-07 19:05:01 浏览: 50
可以使用 python-docx 库将 HTML 转换为 Word 文档。以下是一个简单的示例:
```python
from docx import Document
from bs4 import BeautifulSoup
# 读取 HTML 文件
with open('input.html', 'r') as f:
html = f.read()
# 使用 BeautifulSoup 解析 HTML
soup = BeautifulSoup(html, 'html.parser')
# 创建新的 Word 文档
doc = Document()
# 遍历 HTML 标签并添加到 Word 文档中
for tag in soup.body.children:
if tag.name == 'p':
doc.add_paragraph(tag.text)
elif tag.name == 'ul':
for li in tag.children:
doc.add_paragraph(li.text, style='List Bullet')
# 保存 Word 文档
doc.save('output.docx')
```
请注意,此示例仅处理 HTML 中的段落(`<p>`)和无序列表(`<ul>`)。如果您需要处理其他 HTML 标签,请相应地修改代码。
相关问题
python实现html转word
可以使用Python的`python-docx`库将HTML转换为Word文档。以下是一个简单的示例代码:
```python
from docx import Document
from docx.shared import Inches
from bs4 import BeautifulSoup
import requests
# 获取HTML内容
url = 'https://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
# 创建Word文档
document = Document()
# 遍历HTML标签并将其转换为Word格式
for tag in soup.find_all():
if tag.name == 'p':
paragraph = document.add_paragraph(tag.text)
elif tag.name == 'img':
response = requests.get(tag['src'], stream=True)
image = response.raw
paragraph = document.add_paragraph()
paragraph.add_run().add_picture(image, width=Inches(4))
elif tag.name == 'h1':
heading = document.add_heading(tag.text, level=1)
elif tag.name == 'h2':
heading = document.add_heading(tag.text, level=2)
elif tag.name == 'h3':
heading = document.add_heading(tag.text, level=3)
# 保存Word文档
document.save('output.docx')
```
这个示例代码仅支持`<p>`、`<img>`、`<h1>`、`<h2>`和`<h3>`标签,并将它们转换为Word文档的段落、图片和标题格式。如果你需要支持其他标签,可以在代码中添加相应的处理逻辑。
python word文档转html
您可以使用Python中的`python-docx`库来读取Word文档,并将其转换为HTML格式。以下是一个简单的示例代码:
```python
from docx import Document
from docx2html import convert
# 打开Word文档
document = Document('example.docx')
# 将文档转换为HTML字符串
html = convert(document)
# 将HTML字符串写入文件
with open('example.html', 'w', encoding='utf-8') as f:
f.write(html)
```
请注意,您需要先安装`python-docx`和`docx2html`库。您可以使用以下命令进行安装:
```
pip install python-docx
pip install docx2html
```
另外,请注意,`docx2html`库可能无法完全保留Word文档的格式和样式。
相关推荐
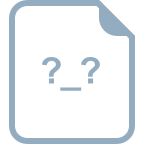
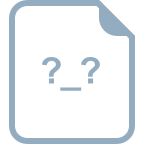
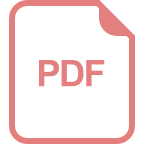
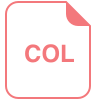











