python代码 word转换html
时间: 2023-12-20 15:03:36 浏览: 134
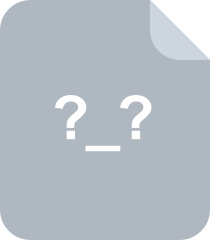
word转换html
这里提供一个简单的Python代码示例,用于将Word文档转换为HTML格式:
```python
import os
import win32com.client
# 创建 Word 应用程序对象
word = win32com.client.Dispatch("Word.Application")
# 打开 Word 文档
doc = word.Documents.Open("path/to/word/document.docx")
# 将 Word 文档保存为 HTML 文件
doc.SaveAs("path/to/html/file.html", FileFormat=8)
# 关闭 Word 文档
doc.Close()
# 退出 Word 应用程序
word.Quit()
```
需要安装 `pywin32` 库,可以使用以下命令进行安装:
```
pip install pywin32
```
请注意,此示例仅适用于 Windows 操作系统。如果您使用的是 Mac 或 Linux 操作系统,则需要使用适当的库和应用程序来执行此转换。
阅读全文
相关推荐
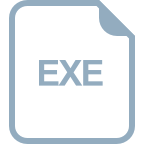



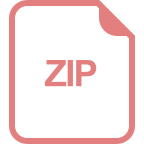
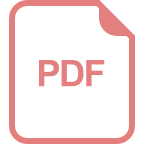
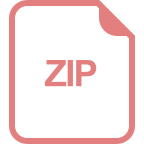
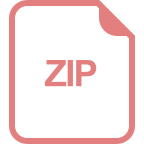
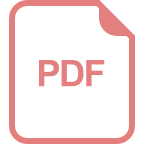
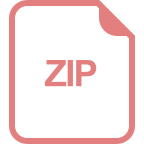
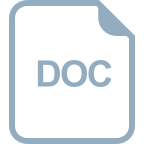





