java如何根据服务器文件路径以及文件名下载任意后缀的文件到程序中
时间: 2023-03-03 09:36:37 浏览: 114
Java可以通过使用标准的Java IO API和网络编程API从服务器上下载文件。以下是一些基本步骤:
1. 创建一个URL对象,使用该对象表示服务器上的文件路径和文件名。
2. 使用URLConnection打开连接并设置HTTP请求的相关属性(例如,使用GET请求方法)。
3. 使用URLConnection.getInputStream()方法获取输入流,这个流可以用于读取服务器上的文件内容。
4. 将输入流写入到程序中,可以使用Java IO API中的文件输出流或其他适当的输出流。
下面是一些示例代码,可以根据您的需要进行修改:
```java
import java.io.*;
import java.net.*;
public class FileDownloader {
public static void main(String[] args) {
String serverFilePath = "http://example.com/path/to/file.jpg"; // 服务器文件路径
String localFilePath = "/path/to/local/file.jpg"; // 下载到本地的文件路径
try {
URL url = new URL(serverFilePath);
URLConnection connection = url.openConnection();
InputStream inputStream = connection.getInputStream();
OutputStream outputStream = new FileOutputStream(localFilePath);
byte[] buffer = new byte[4096];
int bytesRead = -1;
while ((bytesRead = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead);
}
outputStream.close();
inputStream.close();
} catch (MalformedURLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
在上面的示例中,我们首先创建一个URL对象来表示服务器文件路径,然后打开URLConnection并获取输入流。接下来,我们使用Java IO API中的FileOutputStream将输入流写入到本地文件中。
请注意,如果服务器需要进行身份验证或使用HTTPS协议,您可能需要在打开URLConnection之前设置其他HTTP请求属性。具体的细节可能会因服务器的配置而有所不同。
阅读全文
相关推荐




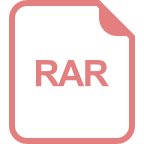










