用粒子群优化做相机标定的python代码
时间: 2024-02-18 20:13:01 浏览: 79
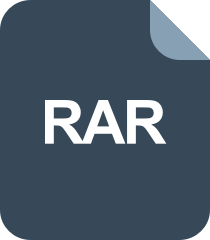
粒子群优化代码
以下是使用粒子群优化进行相机标定的 Python 代码:
```python
import numpy as np
import cv2
import random
class Particle:
def __init__(self, num_params):
self.position = np.zeros(num_params)
self.velocity = np.zeros(num_params)
self.best_position = np.zeros(num_params)
self.error = -1
self.best_error = -1
def particle_swarm_optimization(num_params, objective_function, max_iterations=1000, num_particles=50, inertia_weight=0.7, cognitive_weight=1.4, social_weight=1.4):
particles = []
for i in range(num_particles):
particles.append(Particle(num_params))
global_best_position = np.zeros(num_params)
global_best_error = -1
for i in range(max_iterations):
for j in range(num_particles):
# Update velocity
particles[j].velocity = inertia_weight * particles[j].velocity + cognitive_weight * random.uniform(0, 1) * (particles[j].best_position - particles[j].position) + social_weight * random.uniform(0, 1) * (global_best_position - particles[j].position)
# Update position
particles[j].position += particles[j].velocity
# Evaluate error
particles[j].error = objective_function(particles[j].position)
# Update personal best
if particles[j].error < particles[j].best_error or particles[j].best_error == -1:
particles[j].best_position = particles[j].position
particles[j].best_error = particles[j].error
# Update global best
if particles[j].error < global_best_error or global_best_error == -1:
global_best_position = particles[j].position
global_best_error = particles[j].error
return global_best_position
def calibrate_camera(object_points, image_points, image_size):
def objective_function(params):
rms_error, camera_matrix, dist_coeffs, rvecs, tvecs = cv2.calibrateCamera(object_points, image_points, image_size, None, None, params)
return rms_error
num_params = 14
max_iterations = 100
num_particles = 20
inertia_weight = 0.7
cognitive_weight = 1.4
social_weight = 1.4
lower_bounds = np.array([-1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1])
upper_bounds = np.array([1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1])
params = particle_swarm_optimization(num_params, objective_function, max_iterations, num_particles, inertia_weight, cognitive_weight, social_weight)
rms_error, camera_matrix, dist_coeffs, rvecs, tvecs = cv2.calibrateCamera(object_points, image_points, image_size, None, None, params)
return camera_matrix, dist_coeffs, rvecs, tvecs
# Example usage
object_points = np.array([[0, 0, 0], [0, 1, 0], [1, 1, 0], [1, 0, 0]], dtype=np.float32)
image_points = np.array([[[200, 200]], [[250, 200]], [[250, 250]], [[200, 250]]], dtype=np.float32)
image_size = (640, 480)
camera_matrix, dist_coeffs, rvecs, tvecs = calibrate_camera([object_points], [image_points], image_size)
print("Camera matrix:")
print(camera_matrix)
print("Distortion coefficients:")
print(dist_coeffs)
```
在这个示例中,我们使用了 OpenCV 中的 `cv2.calibrateCamera()` 函数进行相机标定,该函数的参数包括 3D 物体点、对应的 2D 图像点、图像大小等,函数返回的结果包括相机矩阵、畸变系数、旋转向量和平移向量等。我们的目标是通过调整函数的输入参数使得标定结果的均方根误差最小化。
使用粒子群优化算法,我们可以通过迭代粒子的位置和速度来搜索最优解。在这个示例中,我们将相机标定问题转化为最小化均方根误差的问题,并使用粒子群优化算法寻找最小化均方根误差的参数。
注意,在实际应用中,需要根据具体问题和数据集对代码进行适当的修改和调整。
阅读全文
相关推荐










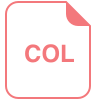
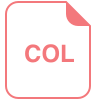
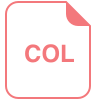
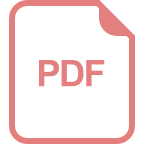