centrality = nx.degree_centrality(graph) nx.set_node_attributes(graph, centrality, 'centrality') degrees = sorted(centrality.items(), key=itemgetter(1), reverse=True) for item in degrees[0:10]: print (item)
时间: 2024-02-29 10:52:57 浏览: 118
这段代码使用了 NetworkX 库中的 `degree_centrality` 函数计算了图中每个节点的度中心性(degree centrality),然后将该结果作为节点属性添加到图中:
```python
centrality = nx.degree_centrality(graph) # 计算度中心性
nx.set_node_attributes(graph, centrality, 'centrality') # 将结果添加到节点属性中
```
接着,代码对节点进行排序并输出前10个节点的度中心性,以便观察最重要的节点:
```python
degrees = sorted(centrality.items(), key=itemgetter(1), reverse=True) # 对节点按度中心性进行排序
for item in degrees[0:10]: # 输出前10个节点
print(item)
```
其中,`sorted` 函数对字典进行排序,`itemgetter` 函数指定以字典的第二个值(即度中心性)进行排序,`reverse=True` 表示按从大到小的顺序排列。最后,代码输出前10个节点的度中心性。
相关问题
nbest_centrality(graph,nx.betweenness_centrality)nbest_centrality(graph,nx.closeness_centrality)nbest_centrality(graph,nx.eigenvector_centrality_numpy)
这些函数都是用于计算图形中节点的中心性指标,并返回前n个中心节点。具体实现可以按照以下步骤:
1. 导入`networkx`模块。
```
import networkx as nx
```
2. 创建一个图形对象,可以从文件中加载或手动添加节点和边缘。
```
G = nx.Graph()
# add nodes and edges to the graph
```
3. 使用所需的中心性指标函数来计算每个节点的中心性值,并将结果存储在字典中。
```
betweenness = nx.betweenness_centrality(G)
closeness = nx.closeness_centrality(G)
eigenvector = nx.eigenvector_centrality_numpy(G)
```
4. 创建一个函数来返回前n个中心节点。该函数可以接受字典、中心性指标函数和n作为参数。
```
def nbest_centrality(graph, centrality_func, n):
centrality = centrality_func(graph)
sorted_nodes = sorted(centrality.items(), key=lambda x: x[1], reverse=True)
return [node[0] for node in sorted_nodes[:n]]
```
5. 调用`nbest_centrality`函数并传递相应的参数来获取前n个中心节点。
```
print(nbest_centrality(G, nx.betweenness_centrality, 5))
print(nbest_centrality(G, nx.closeness_centrality, 5))
print(nbest_centrality(G, nx.eigenvector_centrality_numpy, 5))
```
在这里,我们假设要返回前5个中心节点。你可以根据需要调整`n`的值来获取更多或更少的中心节点。
def centrality(G): #计算度中心性,降序 dc = nx.algorithms.centrality.degree_centrality(G) return sorted(dc.items(), key=lambda x: x[1],reverse = True)啥意思
这段代码实现了计算一个图 G 的节点度中心性,并按照从大到小的顺序返回每个节点及其度中心性。具体解释如下:
- `dc = nx.algorithms.centrality.degree_centrality(G)` 计算图 G 中每个节点的度中心性,并将结果存储在字典 dc 中。
- `sorted(dc.items(), key=lambda x: x[1],reverse = True)` 将字典 dc 中的每个键值对按照值从大到小的顺序排序,并返回一个列表。其中,`key=lambda x: x[1]` 表示按照字典中的值进行排序,`reverse=True` 表示降序排列。
- `return` 返回排序后的列表。
因此,调用 `centrality(G)` 函数可以得到一个按照度中心性从大到小排列的节点列表。
阅读全文
相关推荐
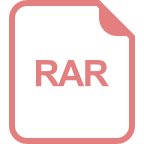
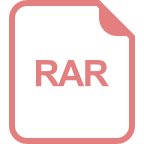
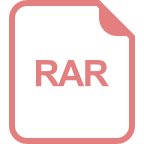
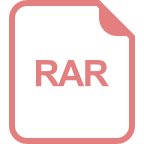








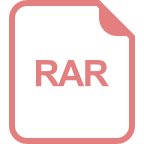

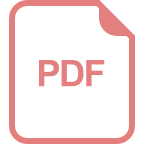