实现二叉树的中序遍历和后序遍历递归和非递归算法
时间: 2023-06-08 21:08:56 浏览: 143
好的,这是一个计算机科学方面的问题,让我来为你解答。
二叉树的中序遍历和后序遍历递归算法可以分别使用以下的递归函数来实现:
中序遍历:
```
void inorder(TreeNode* root) {
if (root == NULL) return;
inorder(root->left);
// 在这里对中间节点进行操作
inorder(root->right);
}
```
后序遍历:
```
void postorder(TreeNode* root) {
if (root == NULL) return;
postorder(root->left);
postorder(root->right);
// 在这里对后续节点进行操作
}
```
而中序遍历和后序遍历的非递归算法则需要使用辅助数据结构,例如栈,来存储节点,然后进行遍历。下面是中序遍历和后续遍历的非递归算法:
中序遍历:
```
void inorder(TreeNode* root) {
stack<TreeNode*> s;
TreeNode* p = root;
while (!s.empty() || p != NULL) {
if (p != NULL) {
s.push(p);
p = p->left;
} else {
p = s.top();
s.pop();
// 在这里对中间节点进行操作
p = p->right;
}
}
}
```
后序遍历:
```
void postorder(TreeNode* root) {
stack<TreeNode*> s;
TreeNode* p = root;
TreeNode* last_visit = NULL;
while (!s.empty() || p != NULL) {
if (p != NULL) {
s.push(p);
p = p->left;
} else {
TreeNode* top_node = s.top();
if (top_node->right != NULL && last_visit != top_node->right) {
p = top_node->right;
} else {
s.pop();
// 在这里对后续节点进行操作
last_visit = top_node;
}
}
}
}
```
希望这些算法对你有所帮助!
阅读全文
相关推荐
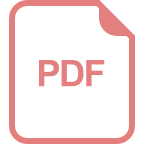
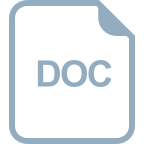









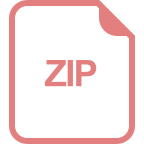
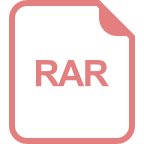

