GAT图分类代码示例,要求使用随机数据(即不用现有数据集)作为测试、训练用,并且图形中的边也要有属性
时间: 2024-02-03 10:11:45 浏览: 142
以下是一个使用PyTorch Geometric实现的简单GAT图分类代码示例,使用随机数据作为训练和测试数据集,同时边也有属性。本例子中图形是一个二分图,包含两种不同的节点类型(type1和type2),以及它们之间的边。我们的目标是使用GAT模型对这个图形进行分类。
```python
import torch
import torch.nn.functional as F
from torch_geometric.datasets import Planetoid
from torch_geometric.nn import GATConv
# 定义一个简单的GAT模型
class GAT(torch.nn.Module):
def __init__(self):
super(GAT, self).__init__()
self.conv1 = GATConv(8, 16, heads=8)
self.conv2 = GATConv(16*8, 2, heads=1)
def forward(self, x, edge_index, edge_attr):
x = F.dropout(x, p=0.6, training=self.training)
x = F.elu(self.conv1(x, edge_index, edge_attr))
x = F.dropout(x, p=0.6, training=self.training)
x = self.conv2(x, edge_index, edge_attr)
return F.log_softmax(x, dim=1)
# 生成随机数据集
x1 = torch.randn(100, 8)
x2 = torch.randn(50, 8)
x = torch.cat([x1, x2], dim=0)
edge_index1 = torch.randint(0, 100, (2, 200))
edge_index2 = torch.randint(100, 150, (2, 200))
edge_index = torch.cat([edge_index1, edge_index2], dim=1)
edge_attr = torch.randn(400, 4)
y1 = torch.zeros(100, dtype=torch.long)
y2 = torch.ones(50, dtype=torch.long)
y = torch.cat([y1, y2], dim=0)
# 将数据转换为PyTorch Geometric格式
from torch_geometric.data import Data
data = Data(x=x, edge_index=edge_index, edge_attr=edge_attr, y=y)
# 分割数据集
from sklearn.model_selection import train_test_split
train_idx, test_idx = train_test_split(range(150), test_size=0.3)
train_mask = torch.zeros(150, dtype=torch.bool)
train_mask[train_idx] = True
test_mask = torch.zeros(150, dtype=torch.bool)
test_mask[test_idx] = True
# 初始化模型并定义优化器
device = torch.device('cuda' if torch.cuda.is_available() else 'cpu')
model = GAT().to(device)
optimizer = torch.optim.Adam(model.parameters(), lr=0.01)
# 训练模型
model.train()
for epoch in range(200):
optimizer.zero_grad()
out = model(data.x.to(device), data.edge_index.to(device), data.edge_attr.to(device))
loss = F.nll_loss(out[train_mask], data.y[train_mask].to(device))
loss.backward()
optimizer.step()
with torch.no_grad():
pred = model(data.x.to(device), data.edge_index.to(device), data.edge_attr.to(device)).argmax(dim=1)
acc = pred[test_mask].eq(data.y[test_mask].to(device)).sum().item() / test_mask.sum().item()
print(f'Epoch: {epoch}, Loss: {loss:.4f}, Test Acc: {acc:.4f}')
```
在这个例子中,我们定义了一个简单的GAT模型,该模型由两个GATConv层组成。我们使用dropout和elu激活函数来提高模型的鲁棒性。我们使用随机数据集(包含100个type1节点和50个type2节点以及它们之间的边)来训练和测试模型。我们使用train_test_split函数将数据集分为训练和测试集。在训练过程中,我们使用Adam优化器和负对数似然损失函数,最终输出测试准确率。
阅读全文
相关推荐
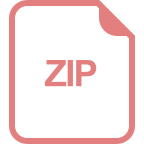


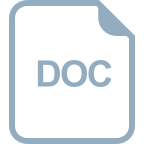












