用C++编写一个vector头文件,给出代码
时间: 2024-02-01 07:13:23 浏览: 81
以下是一个简单的vector头文件的示例代码:
```cpp
#ifndef VECTOR_H
#define VECTOR_H
template<typename T>
class Vector {
private:
T* data;
int size;
int capacity;
void resize(int newCapacity) {
T* newData = new T[newCapacity];
for (int i = 0; i < size; i++) {
newData[i] = data[i];
}
delete[] data;
data = newData;
capacity = newCapacity;
}
public:
Vector() : data(nullptr), size(0), capacity(0) {}
Vector(const Vector<T>& other) : data(nullptr), size(0), capacity(0) {
*this = other;
}
~Vector() {
delete[] data;
}
Vector<T>& operator=(const Vector<T>& other) {
if (this == &other) {
return *this;
}
delete[] data;
data = new T[other.capacity];
for (int i = 0; i < other.size; i++) {
data[i] = other.data[i];
}
size = other.size;
capacity = other.capacity;
return *this;
}
void push_back(const T& value) {
if (size == capacity) {
int newCapacity = (capacity == 0 ? 1 : capacity * 2);
resize(newCapacity);
}
data[size++] = value;
}
void pop_back() {
if (size > 0) {
size--;
}
}
int getSize() const {
return size;
}
T& operator[](int index) {
return data[index];
}
const T& operator[](int index) const {
return data[index];
}
};
#endif
```
该vector类支持动态增长,能够自动调整容量以适应元素的数量。它可以存储任何类型的元素,并提供常规的vector操作,如push_back、pop_back、operator[]等等。
阅读全文
相关推荐
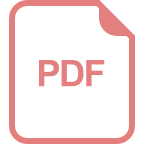

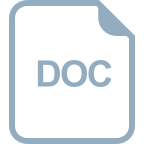
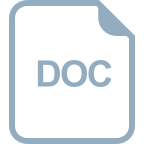
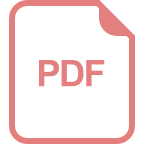
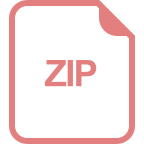
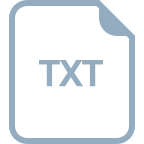
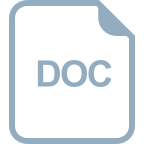
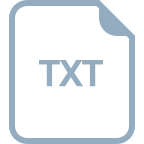
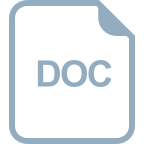
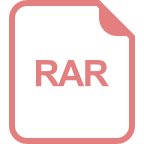
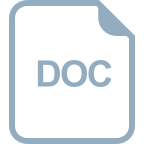
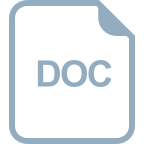
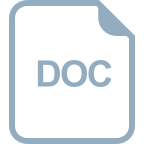
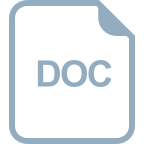

