使用c语言编写衣服销售管理系统 问题描述:衣服信息包括编号、品牌、款式、颜色、库存量、进货价格、销售价格、付款方式等; 付款方式包括现金和刷卡。 功能要求: (1)各种衣服信息需要利用文件的形式进行存储。 (2)输入功能:可以从键盘或文件中一次性输入多条信息; (3)输出功能:可将入库、出库等信息打印输出或显示; (4)查找功能:根据衣服编号、牌子、价格等查找出所需信息; (5)增加、删除、修改功能:; (6)统计功能:完成入库、出库、库存等信息的统计功能。 (7)要求能通过文件保存信息,程序支持信息导入和保存
时间: 2023-07-04 07:26:29 浏览: 58
好的,这是一个比较完整的衣服销售管理系统,需要使用C语言进行编写。下面是一个可能的实现方式:
1. 定义衣服信息结构体
```c
struct Clothes {
char id[10]; // 编号
char brand[20]; // 品牌
char style[20]; // 款式
char color[10]; // 颜色
int stock; // 库存量
float purchase_price; // 进货价格
float sale_price; // 销售价格
char payment_method[10]; // 付款方式
};
```
2. 定义函数实现各种功能
```c
// 读取衣服信息
void read_clothes(struct Clothes *clothes) {
printf("请输入编号:");
scanf("%s", clothes->id);
printf("请输入品牌:");
scanf("%s", clothes->brand);
printf("请输入款式:");
scanf("%s", clothes->style);
printf("请输入颜色:");
scanf("%s", clothes->color);
printf("请输入库存量:");
scanf("%d", &clothes->stock);
printf("请输入进货价格:");
scanf("%f", &clothes->purchase_price);
printf("请输入销售价格:");
scanf("%f", &clothes->sale_price);
printf("请输入付款方式(现金/刷卡):");
scanf("%s", clothes->payment_method);
}
// 打印衣服信息
void print_clothes(struct Clothes clothes) {
printf("编号:%s,品牌:%s,款式:%s,颜色:%s,库存量:%d,进货价格:%.2f,销售价格:%.2f,付款方式:%s\n",
clothes.id, clothes.brand, clothes.style, clothes.color, clothes.stock, clothes.purchase_price, clothes.sale_price, clothes.payment_method);
}
// 保存衣服信息到文件
void save_clothes_to_file(struct Clothes *clothes, int count) {
FILE *fp = fopen("clothes.txt", "w");
for (int i = 0; i < count; i++) {
fprintf(fp, "%s %s %s %s %d %.2f %.2f %s\n",
clothes[i].id, clothes[i].brand, clothes[i].style, clothes[i].color,
clothes[i].stock, clothes[i].purchase_price, clothes[i].sale_price, clothes[i].payment_method);
}
fclose(fp);
}
// 从文件中读取衣服信息
int read_clothes_from_file(struct Clothes *clothes) {
FILE *fp = fopen("clothes.txt", "r");
if (fp == NULL) {
return 0;
}
int count = 0;
while (fscanf(fp, "%s %s %s %s %d %f %f %s",
clothes[count].id, clothes[count].brand, clothes[count].style, clothes[count].color,
&clothes[count].stock, &clothes[count].purchase_price, &clothes[count].sale_price, clothes[count].payment_method) != EOF) {
count++;
}
fclose(fp);
return count;
}
// 按编号查找衣服信息
void find_clothes_by_id(struct Clothes *clothes, int count) {
char id[10];
printf("请输入编号:");
scanf("%s", id);
for (int i = 0; i < count; i++) {
if (strcmp(clothes[i].id, id) == 0) {
print_clothes(clothes[i]);
return;
}
}
printf("没有找到该编号的衣服!\n");
}
// 按品牌查找衣服信息
void find_clothes_by_brand(struct Clothes *clothes, int count) {
char brand[20];
printf("请输入品牌:");
scanf("%s", brand);
for (int i = 0; i < count; i++) {
if (strcmp(clothes[i].brand, brand) == 0) {
print_clothes(clothes[i]);
}
}
}
// 按价格查找衣服信息
void find_clothes_by_price(struct Clothes *clothes, int count) {
float price;
printf("请输入价格:");
scanf("%f", &price);
for (int i = 0; i < count; i++) {
if (clothes[i].sale_price == price) {
print_clothes(clothes[i]);
}
}
}
// 增加衣服信息
void add_clothes(struct Clothes *clothes, int *count) {
read_clothes(&clothes[*count]);
(*count)++;
save_clothes_to_file(clothes, *count);
}
// 删除衣服信息
void delete_clothes(struct Clothes *clothes, int *count) {
char id[10];
printf("请输入编号:");
scanf("%s", id);
for (int i = 0; i < *count; i++) {
if (strcmp(clothes[i].id, id) == 0) {
for (int j = i; j < *count - 1; j++) {
clothes[j] = clothes[j + 1];
}
(*count)--;
save_clothes_to_file(clothes, *count);
printf("删除成功!\n");
return;
}
}
printf("没有找到该编号的衣服!\n");
}
// 修改衣服信息
void modify_clothes(struct Clothes *clothes, int count) {
char id[10];
printf("请输入编号:");
scanf("%s", id);
for (int i = 0; i < count; i++) {
if (strcmp(clothes[i].id, id) == 0) {
printf("请输入新的品牌:");
scanf("%s", clothes[i].brand);
printf("请输入新的款式:");
scanf("%s", clothes[i].style);
printf("请输入新的颜色:");
scanf("%s", clothes[i].color);
printf("请输入新的库存量:");
scanf("%d", &clothes[i].stock);
printf("请输入新的进货价格:");
scanf("%f", &clothes[i].purchase_price);
printf("请输入新的销售价格:");
scanf("%f", &clothes[i].sale_price);
printf("请输入新的付款方式(现金/刷卡):");
scanf("%s", clothes[i].payment_method);
save_clothes_to_file(clothes, count);
printf("修改成功!\n");
return;
}
}
printf("没有找到该编号的衣服!\n");
}
// 统计衣服信息
void statistics_clothes(struct Clothes *clothes, int count) {
int total_stock = 0;
float total_purchase_price = 0.0f, total_sale_price = 0.0f;
for (int i = 0; i < count; i++) {
total_stock += clothes[i].stock;
total_purchase_price += clothes[i].purchase_price;
total_sale_price += clothes[i].sale_price;
}
printf("总库存量:%d,总进货价格:%.2f,总销售价格:%.2f\n", total_stock, total_purchase_price, total_sale_price);
}
```
3. 编写主函数
```c
int main() {
struct Clothes clothes[100];
int count = read_clothes_from_file(clothes);
int choice;
while (1) {
printf("请选择功能:\n");
printf("1. 输入衣服信息\n");
printf("2. 显示所有衣服信息\n");
printf("3. 按编号查找衣服信息\n");
printf("4. 按品牌查找衣服信息\n");
printf("5. 按价格查找衣服信息\n");
printf("6. 增加衣服信息\n");
printf("7. 删除衣服信息\n");
printf("8. 修改衣服信息\n");
printf("9. 统计衣服信息\n");
printf("0. 退出程序\n");
scanf("%d", &choice);
switch (choice) {
case 0:
return 0;
case 1:
printf("请输入衣服信息(编号 品牌 款式 颜色 库存量 进货价格 销售价格 付款方式):\n");
for (int i = 0; i < 100; i++) {
read_clothes(&clothes[count]);
count++;
printf("是否继续输入?(Y/N):");
char c;
scanf(" %c", &c);
if (c == 'N' || c == 'n') {
break;
}
}
save_clothes_to_file(clothes, count);
break;
case 2:
printf("所有衣服信息如下:\n");
for (int i = 0; i < count; i++) {
print_clothes(clothes[i]);
}
break;
case 3:
find_clothes_by_id(clothes, count);
break;
case 4:
find_clothes_by_brand(clothes, count);
break;
case 5:
find_clothes_by_price(clothes, count);
break;
case 6:
add_clothes(clothes, &count);
break;
case 7:
delete_clothes(clothes, &count);
break;
case 8:
modify_clothes(clothes, count);
break;
case 9:
statistics_clothes(clothes, count);
break;
default:
printf("无效的选项,请重新选择!\n");
}
}
}
```
这是一个简单的衣服销售管理系统实现,您可以根据需要进行修改和优化。
相关推荐
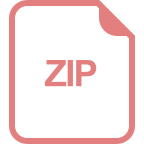
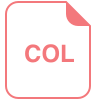
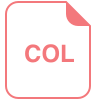
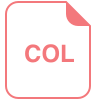
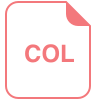
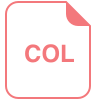









