使用C语言编写一个宠物信息管理系统
时间: 2023-03-22 15:01:00 浏览: 320
恩,我可以给你一些建议。首先,可以先定义需要的宠物信息字段,比如宠物的名字、年龄、性别等等,然后可以使用C语言的结构体数组来存储宠物信息,并使用函数将各种操作封装起来,比如添加宠物信息、查看宠物信息等等。最后,可以建立一个宠物信息管理系统的主函数,根据用户的输入实现不同的功能。
相关问题
使用C语言编写一个宠物信息管理系统的代码
好的,以下是使用C语言编写的一个简单宠物信息管理系统的代码:
```c
#include <stdio.h>
#include <string.h>
#define MAX_PETS 100
struct pet {
char name[50];
int age;
char type[20];
};
struct pet pet_list[MAX_PETS];
int num_pets = 0;
void add_pet() {
struct pet new_pet;
printf("请输入宠物名字:");
scanf("%s", new_pet.name);
printf("请输入宠物年龄:");
scanf("%d", &new_pet.age);
printf("请输入宠物种类:");
scanf("%s", new_pet.type);
pet_list[num_pets] = new_pet;
num_pets++;
}
void list_pets() {
printf("宠物信息列表:\n");
for (int i = 0; i < num_pets; i++) {
printf("宠物 #%d:\n", i+1);
printf("\t名字:%s\n", pet_list[i].name);
printf("\t年龄:%d\n", pet_list[i].age);
printf("\t种类:%s\n", pet_list[i].type);
}
}
int main() {
int choice;
while (1) {
printf("\n请选择要进行的操作:\n");
printf("1. 添加宠物信息\n");
printf("2. 列出所有宠物信息\n");
printf("3. 退出\n");
printf("您的选择:");
scanf("%d", &choice);
switch (choice) {
case 1:
add_pet();
break;
case 2:
list_pets();
break;
case 3:
printf("谢谢使用,再见!\n");
return 0;
default:
printf("无效的选择,请重新选择!\n");
}
}
}
```
这个程序实现了一个简单的宠物信息管理系统,可以添加宠物信息和列出所有宠物信息。用户可以根据提示进行操作。请注意,这只是一个简单的实现,没有进行错误处理和持久化存储。
使用C语言编写一个简单的宠物信息管理系统代码,可以实现宠物信息录入和删除
好的,下面是一个简单的宠物信息管理系统代码,使用C语言编写,可以实现宠物信息录入和删除的功能:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_PETS 100
typedef struct {
char name[20];
int age;
char breed[20];
} Pet;
Pet pets[MAX_PETS];
int num_pets = 0;
void add_pet() {
if (num_pets == MAX_PETS) {
printf("Sorry, the database is full and cannot add more pets.\n");
return;
}
Pet new_pet;
printf("Enter the name of the pet: ");
scanf("%s", new_pet.name);
printf("Enter the age of the pet: ");
scanf("%d", &new_pet.age);
printf("Enter the breed of the pet: ");
scanf("%s", new_pet.breed);
pets[num_pets] = new_pet;
num_pets++;
printf("The pet has been added to the database.\n");
}
void remove_pet() {
if (num_pets == 0) {
printf("Sorry, the database is empty and cannot remove any pets.\n");
return;
}
char name[20];
printf("Enter the name of the pet to remove: ");
scanf("%s", name);
int index = -1;
for (int i = 0; i < num_pets; i++) {
if (strcmp(pets[i].name, name) == 0) {
index = i;
break;
}
}
if (index == -1) {
printf("Sorry, the pet with name %s cannot be found in the database.\n", name);
return;
}
for (int i = index; i < num_pets - 1; i++) {
pets[i] = pets[i + 1];
}
num_pets--;
printf("The pet with name %s has been removed from the database.\n", name);
}
int main() {
int choice;
do {
printf("\n\n");
printf("1. Add a pet\n");
printf("2. Remove a pet\n");
printf("3. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
add_pet();
break;
case 2:
remove_pet();
break;
case 3:
printf("Goodbye!\n");
break;
default:
printf("Invalid choice. Please enter a valid choice.\n");
break;
}
} while (choice != 3);
return 0;
}
```
这个程序中,我们使用了一个结构体 `Pet` 来存储宠物的信息,包括名字、年龄和品种。我们使用一个数组 `pets` 来存储所有的宠物信息,其中 `num_pets` 记录了当前已经录入的宠物数量。程序提供了两个功能,分别是添加宠物和删除宠物。在添加宠物时,程序会提示用户输入宠物的名字、年龄和品种,并将新的宠物信息存储到数组 `pets` 中。在删除宠物时,程序会提示用户输入要删除的宠物的名字,并在数组 `pets` 中查找
阅读全文
相关推荐
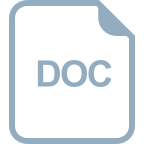
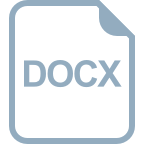
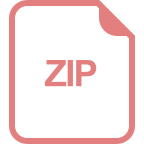












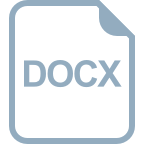
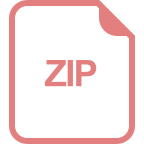