超市购物管理功能python代码
时间: 2023-07-22 07:56:46 浏览: 34
下面是一个简单示例的超市购物管理功能的Python代码:
```python
class Product:
def __init__(self, name, price):
self.name = name
self.price = price
class ShoppingCart:
def __init__(self):
self.items = []
def add_item(self, product, quantity):
self.items.append((product, quantity))
def remove_item(self, product):
for item in self.items:
if item[0].name == product.name:
self.items.remove(item)
break
def calculate_total(self):
total = 0
for item in self.items:
total += item[0].price * item[1]
return total
# 创建商品对象
product1 = Product("Apple", 2.99)
product2 = Product("Banana", 1.99)
product3 = Product("Orange", 3.49)
# 创建购物车对象
cart = ShoppingCart()
# 添加商品到购物车
cart.add_item(product1, 3)
cart.add_item(product2, 2)
cart.add_item(product3, 1)
# 计算购物车总价
total_price = cart.calculate_total()
print(f"Total price: ${total_price}")
# 从购物车中移除商品
cart.remove_item(product2)
# 再次计算购物车总价
total_price = cart.calculate_total()
print(f"Total price after removing item: ${total_price}")
```
这段代码创建了两个类,`Product`表示商品,包含名称和价格两个属性;`ShoppingCart`表示购物车,包含一个保存商品和数量的列表,以及添加、移除和计算总价等方法。
在示例中,创建了三个商品对象,并将它们添加到购物车中。然后计算购物车的总价,并输出结果。接着从购物车中移除了一个商品,再次计算总价并输出结果。
这只是一个简单的示例代码,你可以根据实际需求进行修改和扩展。比如可以添加更多的商品属性和方法,实现更复杂的购物逻辑,或者将数据保存到数据库中等等。
希望这个示例能帮助你入门超市购物管理功能的开发。如果你有更具体的问题或需求,可以进一步提问。
相关推荐
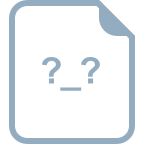
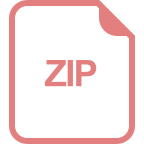
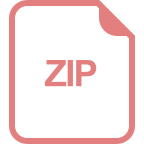














