医学区域生长分割代码实现
时间: 2023-09-24 22:11:01 浏览: 51
医学图像的区域生长分割是一种基于像素相似性的图像分割方法,常用于医学图像中的病变区域分割。下面给出一个基于Python的实现代码示例:
```python
import numpy as np
import cv2
def region_grow(img, seed, threshold):
"""
区域生长分割函数
:param img: 待分割图像
:param seed: 种子点
:param threshold: 阈值
:return: 分割后的二值图像
"""
h, w = img.shape
output = np.zeros((h, w), dtype=np.uint8)
output[seed] = 255
neighbors = [(0, 1), (0, -1), (1, 0), (-1, 0)] # 定义4邻域
while True:
old_points = np.argwhere(output == 255)
new_points = []
for point in old_points:
for neighbor in neighbors:
neighbor_point = tuple(point + neighbor)
if neighbor_point[0] >= h or neighbor_point[0] < 0 \
or neighbor_point[1] >= w or neighbor_point[1] < 0:
continue
if output[neighbor_point] == 0 and abs(img[neighbor_point] - img[point]) < threshold:
output[neighbor_point] = 255
new_points.append(neighbor_point)
if len(new_points) == 0:
break
for point in new_points:
output[point] = 255
return output
if __name__ == '__main__':
img = cv2.imread('test.jpg', cv2.IMREAD_GRAYSCALE) # 读取灰度图像
seed = (100, 100) # 种子点
threshold = 10 # 阈值
output = region_grow(img, seed, threshold)
cv2.imshow('output', output)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在代码中,首先定义了一个区域生长分割函数`region_grow()`,该函数接收一个灰度图像、一个种子点和一个阈值作为输入,返回一个分割后的二值图像。在函数内部,首先初始化输出图像,将种子点标记为前景,然后不断扩展前景像素,直到无法再扩展为止。具体地,对于前景像素的每个4邻域像素,如果该像素未被标记且与前景像素的像素值差小于阈值,则将其标记为前景像素,并将其加入新的前景像素集合中。最后返回分割后的二值图像。
在主函数中,读入待分割的灰度图像,以及定义种子点和阈值,然后调用`region_grow()`函数进行分割,并显示分割后的结果。
相关推荐
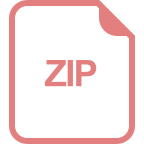
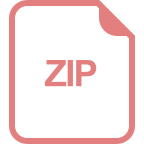
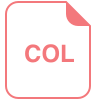
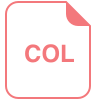
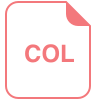











