对一张医学图像,利用python程序,实现基于最大类间方差算法进行图像分割,实现基于迭代阈值算法的图像分割,实现基于区域生长法的左肺分割
时间: 2024-05-06 19:15:20 浏览: 146
好的,我分别介绍一下这三种图像分割算法的实现。
1. 基于最大类间方差算法的图像分割
最大类间方差算法可以用于灰度图像的分割,其基本思想是通过寻找灰度值分割后两个类间方差最大的分割点来进行图像分割。
代码实现如下:
```python
import cv2
import numpy as np
# 读取灰度图像
img = cv2.imread('test.jpg', 0)
# 计算直方图
hist, bins = np.histogram(img, 256, [0, 256])
# 归一化直方图
hist_norm = hist.astype('float32') / img.size
# 初始化类间方差和最大值
inter_class_variance = np.zeros(256)
max_inter_class_variance = 0
best_threshold = 0
# 计算类间方差
for threshold in range(256):
# 计算背景和前景的像素占比
w0 = np.sum(hist_norm[:threshold])
w1 = 1 - w0
# 计算背景和前景的均值
u0 = np.sum(np.arange(threshold) * hist_norm[:threshold]) / w0 if w0 > 0 else 0
u1 = np.sum(np.arange(threshold, 256) * hist_norm[threshold:]) / w1 if w1 > 0 else 0
# 计算类间方差
inter_class_variance[threshold] = w0 * w1 * (u0 - u1) ** 2
# 更新最大类间方差和最佳阈值
if inter_class_variance[threshold] > max_inter_class_variance:
max_inter_class_variance = inter_class_variance[threshold]
best_threshold = threshold
# 二值化图像
img_binary = cv2.threshold(img, best_threshold, 255, cv2.THRESH_BINARY)[1]
# 显示结果
cv2.imshow('Original Image', img)
cv2.imshow('Binary Image', img_binary)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
2. 基于迭代阈值算法的图像分割
迭代阈值算法也是一种基于阈值的图像分割方法,其基本思想是通过不断调整阈值来达到最佳分割效果。
代码实现如下:
```python
import cv2
import numpy as np
# 读取灰度图像
img = cv2.imread('test.jpg', 0)
# 初始化阈值
threshold = 127
# 不断调整阈值,直到稳定
while True:
# 计算背景和前景的像素占比
w0 = np.sum(img <= threshold)
w1 = np.sum(img > threshold)
# 计算背景和前景的均值
u0 = np.mean(img[img <= threshold])
u1 = np.mean(img[img > threshold])
# 更新阈值
new_threshold = int((u0 + u1) / 2)
# 判断是否稳定
if new_threshold == threshold:
break
threshold = new_threshold
# 二值化图像
img_binary = cv2.threshold(img, threshold, 255, cv2.THRESH_BINARY)[1]
# 显示结果
cv2.imshow('Original Image', img)
cv2.imshow('Binary Image', img_binary)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
3. 基于区域生长法的左肺分割
区域生长法是一种基于像素相似性的图像分割方法,其基本思想是从种子点开始,将与种子点像素相似的像素点逐步加入到同一个区域中,直到整个区域被分割出来。
代码实现如下:
```python
import cv2
import numpy as np
# 读取灰度图像
img = cv2.imread('test.jpg', 0)
# 初始化种子点
seed_point = (100, 100)
# 初始化阈值
threshold = 10
# 创建空的掩膜图像
mask = np.zeros_like(img)
# 区域生长法
def region_growing(img, seed_point, threshold, mask):
# 获取图像尺寸
height, width = img.shape
# 复制掩膜图像
mask_copy = mask.copy()
# 初始化队列
queue = []
queue.append(seed_point)
# 循环直到队列为空
while len(queue) > 0:
# 取出队首像素点
current_point = queue.pop(0)
# 获取像素值
current_value = img[current_point[1], current_point[0]]
# 遍历周围8个像素点
for i in range(-1, 2):
for j in range(-1, 2):
# 忽略越界的像素点
if (current_point[1] + i < 0 or current_point[1] + i >= height or
current_point[0] + j < 0 or current_point[0] + j >= width):
continue
# 忽略已经标记过的像素点
if mask[current_point[1] + i, current_point[0] + j] == 255:
continue
# 计算当前像素点和种子点的像素值差
diff = abs(img[current_point[1] + i, current_point[0] + j] - current_value)
# 如果像素值差小于阈值,则加入队列
if diff < threshold:
queue.append((current_point[0] + j, current_point[1] + i))
# 标记像素点
mask_copy[current_point[1] + i, current_point[0] + j] = 255
return mask_copy
# 进行区域生长分割
mask = region_growing(img, seed_point, threshold, mask)
# 显示结果
cv2.imshow('Original Image', img)
cv2.imshow('Mask', mask)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
以上是三种常见的图像分割算法的python实现,希望能对你有所帮助。
阅读全文
相关推荐
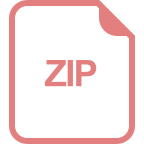
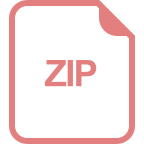
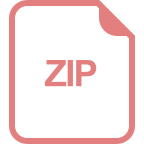
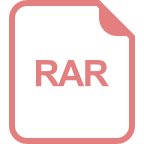
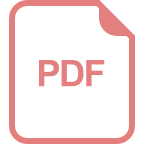
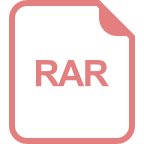
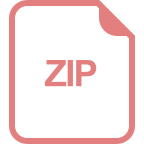
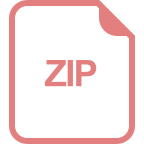
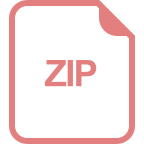
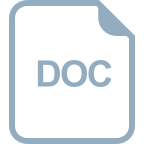
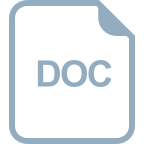
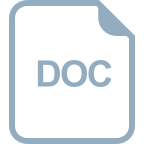
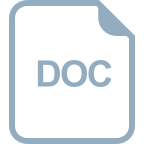
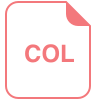
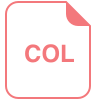
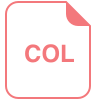
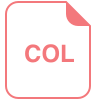