是的
时间: 2024-02-17 10:02:36 浏览: 29
好的,以下是按照您提供的需求编写的Java 7示例代码:
```
import java.time.LocalDate;
import java.time.temporal.ChronoUnit;
import java.util.ArrayList;
import java.util.List;
public class RentContract {
private LocalDate startDate;
private LocalDate endDate;
private LocalDate rentFreeStartDate;
private LocalDate rentFreeEndDate;
private double area;
private double unitPrice;
private int basePeriod;
private int increasePeriod;
private double increaseRate;
public RentContract(LocalDate startDate, LocalDate endDate, LocalDate rentFreeStartDate, LocalDate rentFreeEndDate, double area, double unitPrice, int basePeriod, int increasePeriod, double increaseRate) {
this.startDate = startDate;
this.endDate = endDate;
this.rentFreeStartDate = rentFreeStartDate;
this.rentFreeEndDate = rentFreeEndDate;
this.area = area;
this.unitPrice = unitPrice;
this.basePeriod = basePeriod;
this.increasePeriod = increasePeriod;
this.increaseRate = increaseRate;
}
public List<RentRecord> calculateRentRecords() {
List<RentRecord> records = new ArrayList<>();
LocalDate currentDate = startDate;
while (currentDate.isBefore(endDate)) {
if (currentDate.isBefore(rentFreeEndDate)) {
records.add(new RentRecord(currentDate, null, null, 0));
currentDate = rentFreeEndDate.plusDays(1);
} else {
LocalDate periodStart = currentDate;
LocalDate periodEnd = periodStart.plusMonths(basePeriod).minusDays(1);
if (periodEnd.isAfter(endDate)) {
periodEnd = endDate;
}
double periodPrice = unitPrice;
int periodMonthCount = (int) ChronoUnit.MONTHS.between(periodStart, periodEnd) + 1;
double periodRent = periodMonthCount * area * periodPrice;
records.add(new RentRecord(currentDate, periodStart, periodEnd, periodRent));
currentDate = periodEnd.plusDays(1);
while (currentDate.isBefore(endDate) && ChronoUnit.MONTHS.between(startDate, currentDate) % increasePeriod == 0) {
periodPrice *= (1 + increaseRate);
periodStart = currentDate;
periodEnd = periodStart.plusMonths(increasePeriod).minusDays(1);
if (periodEnd.isAfter(endDate)) {
periodEnd = endDate;
}
periodMonthCount = (int) ChronoUnit.MONTHS.between(periodStart, periodEnd) + 1;
periodRent = periodMonthCount * area * periodPrice;
records.add(new RentRecord(currentDate, periodStart, periodEnd, periodRent));
currentDate = periodEnd.plusDays(1);
}
}
}
return records;
}
public static void main(String[] args) {
RentContract contract = new RentContract(
LocalDate.of(2023, 1, 1),
LocalDate.of(2026, 1, 1),
LocalDate.of(2023, 1, 1),
LocalDate.of(2023, 1, 31),
100,
20,
12,
12,
0.06
);
List<RentRecord> records = contract.calculateRentRecords();
System.out.println("收款日期\t\t租期起始日期\t租期终止日期\t\t租期单价\t租期(月数)\t租金");
for (RentRecord record : records) {
System.out.println(record.getDueDate() + "\t" + record.getPeriodStartDate() + "\t" + record.getPeriodEndDate() + "\t" + contract.unitPrice + "\t" + (int) ChronoUnit.MONTHS.between(record.getPeriodStartDate(), record.getPeriodEndDate()) + "\t\t" + record.getRent());
}
}
}
class RentRecord {
private LocalDate dueDate;
private LocalDate periodStartDate;
private LocalDate periodEndDate;
private double rent;
public RentRecord(LocalDate dueDate, LocalDate periodStartDate, LocalDate periodEndDate, double rent) {
this.dueDate = dueDate;
this.periodStartDate = periodStartDate;
this.periodEndDate = periodEndDate;
this.rent = rent;
}
public LocalDate getDueDate() {
return dueDate;
}
public LocalDate getPeriodStartDate() {
return periodStartDate;
}
public LocalDate getPeriodEndDate() {
return periodEndDate;
}
public double getRent() {
return rent;
}
}
```
上面的代码中,`RentContract`类表示租赁合同,包含合同起始日期、合同结束日期、免租起始日期、免租结束日期、计费面积、月租单价、基本租期(月数)、递增周期(月数)和递增率等属性。`calculateRentRecords`方法用于计算合同期内的租金计划列表,返回一个`RentRecord`对象的列表。`RentRecord`类表示租金记录,包含收款日期、租期起始日期、租期终止日期和租金等属性。
在`main`方法中,我们创建了一个租赁合同对象,并调用`calculateRentRecords`方法计算租金计划列表。最后,我们遍历租金计划列表,依次输出每条记录的收款日期、租期起始日期、租期终止日期、租期单价、租期(月数)和租金。
请注意,由于您没有提供具体的计算方式,因此我们在示例代码中采用了一个可能的计算方式,即每3个月计算一次租金并按3个月收一次款。如果您需要更改计算方式,请修改`calculateRentRecords`方法中的代码。
相关推荐
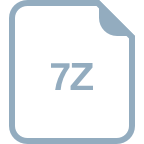
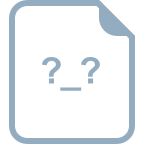















