基于MSP430F49单片机的实时时钟 (1)使用实时时钟芯片DS1302; (2)用1602显示:年、月、日、时、分、秒: (3)可调整时间,设置闹钟。的软件设计
时间: 2023-06-26 11:06:56 浏览: 48
这里给出一个基于MSP430F49单片机的实时时钟软件设计方案:
1. 硬件接线:
将DS1302实时时钟芯片与MSP430F49单片机连接,具体接线方式如下:
DS1302芯片引脚 | 连接的MSP430F49引脚
---|---
RST | P4.4
DAT | P4.5
CLK | P4.6
1602液晶显示屏连接方式如下:
1602液晶显示屏引脚 | 连接的MSP430F49引脚
---|---
RS | P1.0
RW | P1.1
E | P1.2
D0-D3 | 不连接
D4 | P3.4
D5 | P3.5
D6 | P3.6
D7 | P3.7
A | +5V
K | GND
2. 软件设计:
(1) DS1302驱动程序
首先需要编写DS1302驱动程序,用于读取和设置DS1302芯片中的时间和闹钟等信息。
```
#include <msp430.h>
#include "DS1302.h"
void DS1302_Init(void)
{
DS1302_RST_OUT;
DS1302_DAT_OUT;
DS1302_CLK_OUT;
DS1302_RST_SET;
}
void DS1302_WriteByte(unsigned char dat)
{
unsigned char i;
for (i = 0; i < 8; i++)
{
if (dat & 0x01)
DS1302_DAT_SET;
else
DS1302_DAT_CLR;
DS1302_CLK_CLR;
dat >>= 1;
DS1302_CLK_SET;
}
}
unsigned char DS1302_ReadByte(void)
{
unsigned char i, dat = 0;
for (i = 0; i < 8; i++)
{
dat >>= 1;
if (DS1302_DAT_IN & 0x01)
dat |= 0x80;
DS1302_CLK_CLR;
DS1302_CLK_SET;
}
return dat;
}
void DS1302_Write(unsigned char cmd, unsigned char dat)
{
DS1302_RST_SET;
DS1302_CLK_CLR;
DS1302_RST_CLR;
DS1302_WriteByte(cmd);
DS1302_WriteByte(dat);
DS1302_RST_SET;
}
unsigned char DS1302_Read(unsigned char cmd)
{
unsigned char dat;
DS1302_RST_SET;
DS1302_CLK_CLR;
DS1302_RST_CLR;
DS1302_WriteByte(cmd);
dat = DS1302_ReadByte();
DS1302_RST_SET;
return dat;
}
void DS1302_SetTime(unsigned char year, unsigned char month, unsigned char day, unsigned char hour, unsigned char minute, unsigned char second)
{
DS1302_Write(0x8e, 0x00);
DS1302_Write(0x80, second);
DS1302_Write(0x82, minute);
DS1302_Write(0x84, hour);
DS1302_Write(0x86, day);
DS1302_Write(0x88, month);
DS1302_Write(0x8c, year);
DS1302_Write(0x8e, 0x80);
}
void DS1302_GetTime(unsigned char *year, unsigned char *month, unsigned char *day, unsigned char *hour, unsigned char *minute, unsigned char *second)
{
*year = DS1302_Read(0x8d);
*month = DS1302_Read(0x89);
*day = DS1302_Read(0x87);
*hour = DS1302_Read(0x85);
*minute = DS1302_Read(0x83);
*second = DS1302_Read(0x81);
}
void DS1302_SetAlarm(unsigned char hour, unsigned char minute)
{
DS1302_Write(0x8e, 0x00);
DS1302_Write(0x90, minute);
DS1302_Write(0x92, hour);
DS1302_Write(0x8e, 0x80);
}
void DS1302_GetAlarm(unsigned char *hour, unsigned char *minute)
{
*minute = DS1302_Read(0x91);
*hour = DS1302_Read(0x93);
}
```
(2) 1602液晶显示程序
接下来编写1602液晶显示程序,用于显示实时时钟的年、月、日、时、分、秒等信息。
```
#include <msp430.h>
#include "LCD1602.h"
void LCD1602_WriteCmd(unsigned char cmd)
{
LCD1602_RS_CLR;
LCD1602_RW_CLR;
__delay_cycles(1000);
LCD1602_E_SET;
__delay_cycles(1000);
P3OUT = (P3OUT & 0x0f) | (cmd & 0xf0);
__delay_cycles(1000);
LCD1602_E_CLR;
__delay_cycles(1000);
LCD1602_E_SET;
__delay_cycles(1000);
P3OUT = (P3OUT & 0x0f) | ((cmd << 4) & 0xf0);
__delay_cycles(1000);
LCD1602_E_CLR;
__delay_cycles(1000);
}
void LCD1602_WriteData(unsigned char dat)
{
LCD1602_RS_SET;
LCD1602_RW_CLR;
__delay_cycles(1000);
LCD1602_E_SET;
__delay_cycles(1000);
P3OUT = (P3OUT & 0x0f) | (dat & 0xf0);
__delay_cycles(1000);
LCD1602_E_CLR;
__delay_cycles(1000);
LCD1602_E_SET;
__delay_cycles(1000);
P3OUT = (P3OUT & 0x0f) | ((dat << 4) & 0xf0);
__delay_cycles(1000);
LCD1602_E_CLR;
__delay_cycles(1000);
}
void LCD1602_Init(void)
{
P3DIR |= 0xf0;
P1DIR |= 0x07;
__delay_cycles(16000);
LCD1602_WriteCmd(0x33);
__delay_cycles(4000);
LCD1602_WriteCmd(0x32);
__delay_cycles(4000);
LCD1602_WriteCmd(0x28);
__delay_cycles(4000);
LCD1602_WriteCmd(0x0c);
__delay_cycles(4000);
LCD1602_WriteCmd(0x06);
__delay_cycles(4000);
LCD1602_WriteCmd(0x01);
__delay_cycles(4000);
}
void LCD1602_Clear(void)
{
LCD1602_WriteCmd(0x01);
__delay_cycles(4000);
}
void LCD1602_SetCursor(unsigned char row, unsigned char col)
{
unsigned char pos;
if (row == 0)
pos = 0x80 + col;
else
pos = 0xc0 + col;
LCD1602_WriteCmd(pos);
__delay_cycles(4000);
}
void LCD1602_PrintChar(unsigned char dat)
{
LCD1602_WriteData(dat);
__delay_cycles(1000);
}
void LCD1602_PrintString(unsigned char *str)
{
while (*str != '\0')
LCD1602_PrintChar(*str++);
}
void LCD1602_PrintNum(unsigned char num)
{
LCD1602_PrintChar(num / 10 + '0');
LCD1602_PrintChar(num % 10 + '0');
}
```
(3) 主程序
最后编写主程序,实现实时时钟的显示和设置闹钟等功能。
```
#include <msp430.h>
#include "DS1302.h"
#include "LCD1602.h"
unsigned char year, month, day, hour, minute, second;
int main(void)
{
WDTCTL = WDTPW | WDTHOLD;
UCSCTL1 |= DCORSEL_5;
UCSCTL2 = FLLD_1 + 255;
UCSCTL3 = SELREF_2;
UCSCTL4 = SELA_2 + SELS_3 + SELM_3;
UCSCTL5 = DIVA_0 + DIVS_0 + DIVM_0;
UCSCTL7 &= ~(XT2OFFG + XT1LFOFFG + DCOFFG);
UCSCTL8 = SMCLKREQEN + MCLKREQEN + ACLKREQEN;
DS1302_Init();
LCD1602_Init();
while (1)
{
DS1302_GetTime(&year, &month, &day, &hour, &minute, &second);
LCD1602_Clear();
LCD1602_SetCursor(0, 0);
LCD1602_PrintString("20");
LCD1602_PrintNum(year);
LCD1602_PrintChar('-');
LCD1602_PrintNum(month);
LCD1602_PrintChar('-');
LCD1602_PrintNum(day);
LCD1602_SetCursor(1, 0);
LCD1602_PrintNum(hour);
LCD1602_PrintChar(':');
LCD1602_PrintNum(minute);
LCD1602_PrintChar(':');
LCD1602_PrintNum(second);
__delay_cycles(1000000);
}
}
```
以上就是基于MSP430F49单片机的实时时钟软件设计方案。
相关推荐
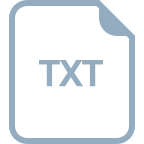














